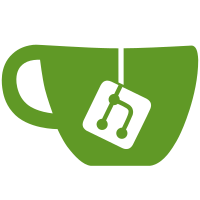
23 changed files with 778 additions and 957 deletions
-
3build/optimize.ts
-
32package.json
-
1149pnpm-lock.yaml
-
2public/serverConfig.json
-
4src/directives/auth/index.ts
-
33src/directives/copy/index.ts
-
27src/directives/elResizeDetector/index.ts
-
3src/directives/index.ts
-
55src/directives/optimize/index.ts
-
11src/layout/components/panel/index.vue
-
19src/layout/components/sidebar/horizontal.vue
-
11src/layout/components/sidebar/mixNav.vue
-
43src/layout/components/sidebar/vertical.vue
-
76src/layout/components/tag/index.vue
-
33src/layout/hooks/useNav.ts
-
12src/layout/index.vue
-
2src/layout/types.ts
-
5src/router/index.ts
-
2src/router/utils.ts
-
1src/store/modules/types.ts
-
12src/utils/mitt.ts
-
93types/global.d.ts
-
105types/router.d.ts
1149
pnpm-lock.yaml
File diff suppressed because it is too large
View File
File diff suppressed because it is too large
View File
@ -0,0 +1,33 @@ |
|||||
|
import { message } from "@/utils/message"; |
||||
|
import { useEventListener } from "@vueuse/core"; |
||||
|
import { copyTextToClipboard } from "@pureadmin/utils"; |
||||
|
import { Directive, type DirectiveBinding } from "vue"; |
||||
|
|
||||
|
interface CopyEl extends HTMLElement { |
||||
|
copyValue: string; |
||||
|
} |
||||
|
|
||||
|
/** 文本复制指令(默认双击复制) */ |
||||
|
export const copy: Directive = { |
||||
|
mounted(el: CopyEl, binding: DirectiveBinding) { |
||||
|
const { value } = binding; |
||||
|
if (value) { |
||||
|
el.copyValue = value; |
||||
|
const arg = binding.arg ?? "dblclick"; |
||||
|
// Register using addEventListener on mounted, and removeEventListener automatically on unmounted
|
||||
|
useEventListener(el, arg, () => { |
||||
|
const success = copyTextToClipboard(el.copyValue); |
||||
|
success |
||||
|
? message("复制成功", { type: "success" }) |
||||
|
: message("复制失败", { type: "error" }); |
||||
|
}); |
||||
|
} else { |
||||
|
throw new Error( |
||||
|
'[Directive: copy]: need value! Like v-copy="modelValue"' |
||||
|
); |
||||
|
} |
||||
|
}, |
||||
|
updated(el: CopyEl, binding: DirectiveBinding) { |
||||
|
el.copyValue = binding.value; |
||||
|
} |
||||
|
}; |
@ -1,27 +0,0 @@ |
|||||
import { Directive, type DirectiveBinding, type VNode } from "vue"; |
|
||||
import elementResizeDetectorMaker from "element-resize-detector"; |
|
||||
import type { Erd } from "element-resize-detector"; |
|
||||
import { emitter } from "@/utils/mitt"; |
|
||||
|
|
||||
const erd: Erd = elementResizeDetectorMaker({ |
|
||||
strategy: "scroll" |
|
||||
}); |
|
||||
|
|
||||
export const resize: Directive = { |
|
||||
mounted(el: HTMLElement, binding?: DirectiveBinding, vnode?: VNode) { |
|
||||
erd.listenTo(el, elem => { |
|
||||
const width = elem.offsetWidth; |
|
||||
const height = elem.offsetHeight; |
|
||||
if (binding?.instance) { |
|
||||
emitter.emit("resize", { detail: { width, height } }); |
|
||||
} else { |
|
||||
vnode.el.dispatchEvent( |
|
||||
new CustomEvent("resize", { detail: { width, height } }) |
|
||||
); |
|
||||
} |
|
||||
}); |
|
||||
}, |
|
||||
unmounted(el: HTMLElement) { |
|
||||
erd.uninstall(el); |
|
||||
} |
|
||||
}; |
|
@ -1,2 +1,3 @@ |
|||||
export * from "./auth"; |
export * from "./auth"; |
||||
export * from "./elResizeDetector"; |
|
||||
|
export * from "./copy"; |
||||
|
export * from "./optimize"; |
@ -0,0 +1,55 @@ |
|||||
|
import { |
||||
|
isFunction, |
||||
|
isObject, |
||||
|
isArray, |
||||
|
debounce, |
||||
|
throttle |
||||
|
} from "@pureadmin/utils"; |
||||
|
import { useEventListener } from "@vueuse/core"; |
||||
|
import { Directive, type DirectiveBinding } from "vue"; |
||||
|
|
||||
|
/** 防抖(v-optimize或v-optimize:debounce)、节流(v-optimize:throttle)指令 */ |
||||
|
export const optimize: Directive = { |
||||
|
mounted(el: HTMLElement, binding: DirectiveBinding) { |
||||
|
const { value } = binding; |
||||
|
const optimizeType = binding.arg ?? "debounce"; |
||||
|
const type = ["debounce", "throttle"].find(t => t === optimizeType); |
||||
|
if (type) { |
||||
|
if (value && value.event && isFunction(value.fn)) { |
||||
|
let params = value?.params; |
||||
|
if (params) { |
||||
|
if (isArray(params) || isObject(params)) { |
||||
|
params = isObject(params) ? Array.of(params) : params; |
||||
|
} else { |
||||
|
throw new Error( |
||||
|
"[Directive: optimize]: `params` must be an array or object" |
||||
|
); |
||||
|
} |
||||
|
} |
||||
|
// Register using addEventListener on mounted, and removeEventListener automatically on unmounted
|
||||
|
useEventListener( |
||||
|
el, |
||||
|
value.event, |
||||
|
type === "debounce" |
||||
|
? debounce( |
||||
|
params ? () => value.fn(...params) : value.fn, |
||||
|
value?.timeout ?? 200, |
||||
|
value?.immediate ?? false |
||||
|
) |
||||
|
: throttle( |
||||
|
params ? () => value.fn(...params) : value.fn, |
||||
|
value?.timeout ?? 1000 |
||||
|
) |
||||
|
); |
||||
|
} else { |
||||
|
throw new Error( |
||||
|
"[Directive: optimize]: `event` and `fn` are required, and `fn` must be a function" |
||||
|
); |
||||
|
} |
||||
|
} else { |
||||
|
throw new Error( |
||||
|
"[Directive: optimize]: only `debounce` and `throttle` are supported" |
||||
|
); |
||||
|
} |
||||
|
} |
||||
|
}; |
@ -1,21 +1,13 @@ |
|||||
import type { Emitter } from "mitt"; |
import type { Emitter } from "mitt"; |
||||
import mitt from "mitt"; |
import mitt from "mitt"; |
||||
|
|
||||
|
/** 全局公共事件需要在此处添加类型 */ |
||||
type Events = { |
type Events = { |
||||
resize: { |
|
||||
detail: { |
|
||||
width: number; |
|
||||
height: number; |
|
||||
}; |
|
||||
}; |
|
||||
openPanel: string; |
openPanel: string; |
||||
tagViewsChange: string; |
tagViewsChange: string; |
||||
tagViewsShowModel: string; |
tagViewsShowModel: string; |
||||
logoChange: boolean; |
logoChange: boolean; |
||||
changLayoutRoute: { |
|
||||
indexPath: string; |
|
||||
parentPath: string; |
|
||||
}; |
|
||||
|
changLayoutRoute: string; |
||||
}; |
}; |
||||
|
|
||||
export const emitter: Emitter<Events> = mitt<Events>(); |
export const emitter: Emitter<Events> = mitt<Events>(); |
@ -0,0 +1,105 @@ |
|||||
|
// 全局路由类型声明
|
||||
|
|
||||
|
import { type RouteComponent, type RouteLocationNormalized } from "vue-router"; |
||||
|
|
||||
|
declare global { |
||||
|
interface ToRouteType extends RouteLocationNormalized { |
||||
|
meta: CustomizeRouteMeta; |
||||
|
} |
||||
|
|
||||
|
/** |
||||
|
* @description 完整子路由的`meta`配置表 |
||||
|
*/ |
||||
|
interface CustomizeRouteMeta { |
||||
|
/** 菜单名称(兼容国际化、非国际化,如何用国际化的写法就必须在根目录的`locales`文件夹下对应添加) `必填` */ |
||||
|
title: string; |
||||
|
/** 菜单图标 `可选` */ |
||||
|
icon?: string | FunctionalComponent | IconifyIcon; |
||||
|
/** 菜单名称右侧的额外图标 */ |
||||
|
extraIcon?: string | FunctionalComponent | IconifyIcon; |
||||
|
/** 是否在菜单中显示(默认`true`)`可选` */ |
||||
|
showLink?: boolean; |
||||
|
/** 是否显示父级菜单 `可选` */ |
||||
|
showParent?: boolean; |
||||
|
/** 页面级别权限设置 `可选` */ |
||||
|
roles?: Array<string>; |
||||
|
/** 按钮级别权限设置 `可选` */ |
||||
|
auths?: Array<string>; |
||||
|
/** 路由组件缓存(开启 `true`、关闭 `false`)`可选` */ |
||||
|
keepAlive?: boolean; |
||||
|
/** 内嵌的`iframe`链接 `可选` */ |
||||
|
frameSrc?: string; |
||||
|
/** `iframe`页是否开启首次加载动画(默认`true`)`可选` */ |
||||
|
frameLoading?: boolean; |
||||
|
/** 页面加载动画(有两种形式,一种直接采用vue内置的`transitions`动画,另一种是使用`animate.css`写进、离场动画)`可选` */ |
||||
|
transition?: { |
||||
|
/** |
||||
|
* @description 当前路由动画效果 |
||||
|
* @see {@link https://next.router.vuejs.org/guide/advanced/transitions.html#transitions}
|
||||
|
* @see animate.css {@link https://animate.style}
|
||||
|
*/ |
||||
|
name?: string; |
||||
|
/** 进场动画 */ |
||||
|
enterTransition?: string; |
||||
|
/** 离场动画 */ |
||||
|
leaveTransition?: string; |
||||
|
}; |
||||
|
// 是否不添加信息到标签页,(默认`false`)
|
||||
|
hiddenTag?: boolean; |
||||
|
/** 动态路由可打开的最大数量 `可选` */ |
||||
|
dynamicLevel?: number; |
||||
|
/** 将某个菜单激活 |
||||
|
* (主要用于通过`query`或`params`传参的路由,当它们通过配置`showLink: false`后不在菜单中显示,就不会有任何菜单高亮, |
||||
|
* 而通过设置`activePath`指定激活菜单即可获得高亮,`activePath`为指定激活菜单的`path`) |
||||
|
*/ |
||||
|
activePath?: string; |
||||
|
} |
||||
|
|
||||
|
/** |
||||
|
* @description 完整子路由配置表 |
||||
|
*/ |
||||
|
interface RouteChildrenConfigsTable { |
||||
|
/** 子路由地址 `必填` */ |
||||
|
path: string; |
||||
|
/** 路由名字(对应不要重复,和当前组件的`name`保持一致)`必填` */ |
||||
|
name?: string; |
||||
|
/** 路由重定向 `可选` */ |
||||
|
redirect?: string; |
||||
|
/** 按需加载组件 `可选` */ |
||||
|
component?: RouteComponent; |
||||
|
meta?: CustomizeRouteMeta; |
||||
|
/** 子路由配置项 */ |
||||
|
children?: Array<RouteChildrenConfigsTable>; |
||||
|
} |
||||
|
|
||||
|
/** |
||||
|
* @description 整体路由配置表(包括完整子路由) |
||||
|
*/ |
||||
|
interface RouteConfigsTable { |
||||
|
/** 路由地址 `必填` */ |
||||
|
path: string; |
||||
|
/** 路由名字(保持唯一)`可选` */ |
||||
|
name?: string; |
||||
|
/** `Layout`组件 `可选` */ |
||||
|
component?: RouteComponent; |
||||
|
/** 路由重定向 `可选` */ |
||||
|
redirect?: string; |
||||
|
meta?: { |
||||
|
/** 菜单名称(兼容国际化、非国际化,如何用国际化的写法就必须在根目录的`locales`文件夹下对应添加)`必填` */ |
||||
|
title: string; |
||||
|
/** 菜单图标 `可选` */ |
||||
|
icon?: string | FunctionalComponent | IconifyIcon; |
||||
|
/** 是否在菜单中显示(默认`true`)`可选` */ |
||||
|
showLink?: boolean; |
||||
|
/** 菜单升序排序,值越高排的越后(只针对顶级路由)`可选` */ |
||||
|
rank?: number; |
||||
|
}; |
||||
|
/** 子路由配置项 */ |
||||
|
children?: Array<RouteChildrenConfigsTable>; |
||||
|
} |
||||
|
} |
||||
|
|
||||
|
// https://router.vuejs.org/zh/guide/advanced/meta.html#typescript
|
||||
|
declare module "vue-router" { |
||||
|
interface RouteMeta extends CustomizeRouteMeta {} |
||||
|
} |
Write
Preview
Loading…
Cancel
Save
Reference in new issue