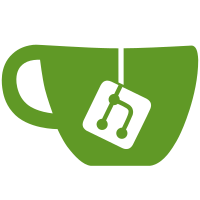
20 changed files with 34 additions and 1375 deletions
-
33locales/en.yaml
-
33locales/zh-CN.yaml
-
2package.json
-
137pnpm-lock.yaml
-
7src/components/ReImageVerify/index.ts
-
85src/components/ReImageVerify/src/hooks.ts
-
46src/components/ReImageVerify/src/index.vue
-
7src/components/ReQrcode/index.ts
-
8src/components/ReQrcode/src/index.scss
-
262src/components/ReQrcode/src/index.tsx
-
12src/store/modules/user.ts
-
4src/style/sidebar.scss
-
106src/views/login/components/phone.vue
-
25src/views/login/components/qrCode.vue
-
189src/views/login/components/regist.vue
-
151src/views/login/components/update.vue
-
102src/views/login/index.vue
-
34src/views/login/utils/enums.ts
-
99src/views/login/utils/rule.ts
-
49src/views/login/utils/verifyCode.ts
@ -1,7 +0,0 @@ |
|||||
import reImageVerify from "./src/index.vue"; |
|
||||
import { withInstall } from "@pureadmin/utils"; |
|
||||
|
|
||||
/** 图形验证码组件 */ |
|
||||
export const ReImageVerify = withInstall(reImageVerify); |
|
||||
|
|
||||
export default ReImageVerify; |
|
@ -1,85 +0,0 @@ |
|||||
import { ref, onMounted } from "vue"; |
|
||||
|
|
||||
/** |
|
||||
* 绘制图形验证码 |
|
||||
* @param width - 图形宽度 |
|
||||
* @param height - 图形高度 |
|
||||
*/ |
|
||||
export const useImageVerify = (width = 120, height = 40) => { |
|
||||
const domRef = ref<HTMLCanvasElement>(); |
|
||||
const imgCode = ref(""); |
|
||||
|
|
||||
function setImgCode(code: string) { |
|
||||
imgCode.value = code; |
|
||||
} |
|
||||
|
|
||||
function getImgCode() { |
|
||||
if (!domRef.value) return; |
|
||||
imgCode.value = draw(domRef.value, width, height); |
|
||||
} |
|
||||
|
|
||||
onMounted(() => { |
|
||||
getImgCode(); |
|
||||
}); |
|
||||
|
|
||||
return { |
|
||||
domRef, |
|
||||
imgCode, |
|
||||
setImgCode, |
|
||||
getImgCode |
|
||||
}; |
|
||||
}; |
|
||||
|
|
||||
function randomNum(min: number, max: number) { |
|
||||
const num = Math.floor(Math.random() * (max - min) + min); |
|
||||
return num; |
|
||||
} |
|
||||
|
|
||||
function randomColor(min: number, max: number) { |
|
||||
const r = randomNum(min, max); |
|
||||
const g = randomNum(min, max); |
|
||||
const b = randomNum(min, max); |
|
||||
return `rgb(${r},${g},${b})`; |
|
||||
} |
|
||||
|
|
||||
function draw(dom: HTMLCanvasElement, width: number, height: number) { |
|
||||
let imgCode = ""; |
|
||||
|
|
||||
const NUMBER_STRING = "0123456789"; |
|
||||
|
|
||||
const ctx = dom.getContext("2d"); |
|
||||
if (!ctx) return imgCode; |
|
||||
|
|
||||
ctx.fillStyle = randomColor(180, 230); |
|
||||
ctx.fillRect(0, 0, width, height); |
|
||||
for (let i = 0; i < 4; i += 1) { |
|
||||
const text = NUMBER_STRING[randomNum(0, NUMBER_STRING.length)]; |
|
||||
imgCode += text; |
|
||||
const fontSize = randomNum(18, 41); |
|
||||
const deg = randomNum(-30, 30); |
|
||||
ctx.font = `${fontSize}px Simhei`; |
|
||||
ctx.textBaseline = "top"; |
|
||||
ctx.fillStyle = randomColor(80, 150); |
|
||||
ctx.save(); |
|
||||
ctx.translate(30 * i + 15, 15); |
|
||||
ctx.rotate((deg * Math.PI) / 180); |
|
||||
ctx.fillText(text, -15 + 5, -15); |
|
||||
ctx.restore(); |
|
||||
} |
|
||||
for (let i = 0; i < 5; i += 1) { |
|
||||
ctx.beginPath(); |
|
||||
ctx.moveTo(randomNum(0, width), randomNum(0, height)); |
|
||||
ctx.lineTo(randomNum(0, width), randomNum(0, height)); |
|
||||
ctx.strokeStyle = randomColor(180, 230); |
|
||||
ctx.closePath(); |
|
||||
ctx.stroke(); |
|
||||
} |
|
||||
for (let i = 0; i < 41; i += 1) { |
|
||||
ctx.beginPath(); |
|
||||
ctx.arc(randomNum(0, width), randomNum(0, height), 1, 0, 2 * Math.PI); |
|
||||
ctx.closePath(); |
|
||||
ctx.fillStyle = randomColor(150, 200); |
|
||||
ctx.fill(); |
|
||||
} |
|
||||
return imgCode; |
|
||||
} |
|
@ -1,46 +0,0 @@ |
|||||
<script setup lang="ts"> |
|
||||
import { watch } from "vue"; |
|
||||
import { useImageVerify } from "./hooks"; |
|
||||
|
|
||||
defineOptions({ |
|
||||
name: "ReImageVerify" |
|
||||
}); |
|
||||
|
|
||||
interface Props { |
|
||||
code?: string; |
|
||||
} |
|
||||
|
|
||||
interface Emits { |
|
||||
(e: "update:code", code: string): void; |
|
||||
} |
|
||||
|
|
||||
const props = withDefaults(defineProps<Props>(), { |
|
||||
code: "" |
|
||||
}); |
|
||||
|
|
||||
const emit = defineEmits<Emits>(); |
|
||||
|
|
||||
const { domRef, imgCode, setImgCode, getImgCode } = useImageVerify(); |
|
||||
|
|
||||
watch( |
|
||||
() => props.code, |
|
||||
newValue => { |
|
||||
setImgCode(newValue); |
|
||||
} |
|
||||
); |
|
||||
watch(imgCode, newValue => { |
|
||||
emit("update:code", newValue); |
|
||||
}); |
|
||||
|
|
||||
defineExpose({ getImgCode }); |
|
||||
</script> |
|
||||
|
|
||||
<template> |
|
||||
<canvas |
|
||||
ref="domRef" |
|
||||
width="120" |
|
||||
height="40" |
|
||||
class="cursor-pointer" |
|
||||
@click="getImgCode" |
|
||||
/> |
|
||||
</template> |
|
@ -1,7 +0,0 @@ |
|||||
import reQrcode from "./src/index"; |
|
||||
import { withInstall } from "@pureadmin/utils"; |
|
||||
|
|
||||
/** 二维码组件 */ |
|
||||
export const ReQrcode = withInstall(reQrcode); |
|
||||
|
|
||||
export default ReQrcode; |
|
@ -1,8 +0,0 @@ |
|||||
.qrcode { |
|
||||
&--disabled { |
|
||||
background: rgba(255, 255, 255, 0.95); |
|
||||
& > div { |
|
||||
transform: translate(-50%, -50%); |
|
||||
} |
|
||||
} |
|
||||
} |
|
@ -1,262 +0,0 @@ |
|||||
import { |
|
||||
ref, |
|
||||
unref, |
|
||||
watch, |
|
||||
nextTick, |
|
||||
computed, |
|
||||
PropType, |
|
||||
defineComponent |
|
||||
} from "vue"; |
|
||||
import "./index.scss"; |
|
||||
import { cloneDeep } from "lodash-unified"; |
|
||||
import { isString } from "@pureadmin/utils"; |
|
||||
import { propTypes } from "/@/utils/propTypes"; |
|
||||
import { IconifyIconOffline } from "../../ReIcon"; |
|
||||
import QRCode, { QRCodeRenderersOptions } from "qrcode"; |
|
||||
|
|
||||
interface QrcodeLogo { |
|
||||
src?: string; |
|
||||
logoSize?: number; |
|
||||
bgColor?: string; |
|
||||
borderSize?: number; |
|
||||
crossOrigin?: string; |
|
||||
borderRadius?: number; |
|
||||
logoRadius?: number; |
|
||||
} |
|
||||
|
|
||||
const props = { |
|
||||
// img 或者 canvas,img不支持logo嵌套
|
|
||||
tag: propTypes.string |
|
||||
.validate((v: string) => ["canvas", "img"].includes(v)) |
|
||||
.def("canvas"), |
|
||||
// 二维码内容
|
|
||||
text: { |
|
||||
type: [String, Array] as PropType<string | Recordable[]>, |
|
||||
default: null |
|
||||
}, |
|
||||
// qrcode.js配置项
|
|
||||
options: { |
|
||||
type: Object as PropType<QRCodeRenderersOptions>, |
|
||||
default: (): QRCodeRenderersOptions => ({}) |
|
||||
}, |
|
||||
// 宽度
|
|
||||
width: propTypes.number.def(200), |
|
||||
// logo
|
|
||||
logo: { |
|
||||
type: [String, Object] as PropType<Partial<QrcodeLogo> | string>, |
|
||||
default: (): QrcodeLogo | string => "" |
|
||||
}, |
|
||||
// 是否过期
|
|
||||
disabled: propTypes.bool.def(false), |
|
||||
// 过期提示内容
|
|
||||
disabledText: propTypes.string.def("") |
|
||||
}; |
|
||||
|
|
||||
export default defineComponent({ |
|
||||
name: "ReQrcode", |
|
||||
props, |
|
||||
emits: ["done", "click", "disabled-click"], |
|
||||
setup(props, { emit }) { |
|
||||
const { toCanvas, toDataURL } = QRCode; |
|
||||
const loading = ref(true); |
|
||||
const wrapRef = ref<Nullable<HTMLCanvasElement | HTMLImageElement>>(null); |
|
||||
const renderText = computed(() => String(props.text)); |
|
||||
const wrapStyle = computed(() => { |
|
||||
return { |
|
||||
width: props.width + "px", |
|
||||
height: props.width + "px" |
|
||||
}; |
|
||||
}); |
|
||||
const initQrcode = async () => { |
|
||||
await nextTick(); |
|
||||
const options = cloneDeep(props.options || {}); |
|
||||
if (props.tag === "canvas") { |
|
||||
// 容错率,默认对内容少的二维码采用高容错率,内容多的二维码采用低容错率
|
|
||||
options.errorCorrectionLevel = |
|
||||
options.errorCorrectionLevel || |
|
||||
getErrorCorrectionLevel(unref(renderText)); |
|
||||
const _width: number = await getOriginWidth(unref(renderText), options); |
|
||||
options.scale = |
|
||||
props.width === 0 ? undefined : (props.width / _width) * 4; |
|
||||
const canvasRef: any = await toCanvas( |
|
||||
unref(wrapRef) as HTMLCanvasElement, |
|
||||
unref(renderText), |
|
||||
options |
|
||||
); |
|
||||
if (props.logo) { |
|
||||
const url = await createLogoCode(canvasRef); |
|
||||
emit("done", url); |
|
||||
loading.value = false; |
|
||||
} else { |
|
||||
emit("done", canvasRef.toDataURL()); |
|
||||
loading.value = false; |
|
||||
} |
|
||||
} else { |
|
||||
const url = await toDataURL(renderText.value, { |
|
||||
errorCorrectionLevel: "H", |
|
||||
width: props.width, |
|
||||
...options |
|
||||
}); |
|
||||
(unref(wrapRef) as HTMLImageElement).src = url; |
|
||||
emit("done", url); |
|
||||
loading.value = false; |
|
||||
} |
|
||||
}; |
|
||||
watch( |
|
||||
() => renderText.value, |
|
||||
val => { |
|
||||
if (!val) return; |
|
||||
initQrcode(); |
|
||||
}, |
|
||||
{ |
|
||||
deep: true, |
|
||||
immediate: true |
|
||||
} |
|
||||
); |
|
||||
const createLogoCode = (canvasRef: HTMLCanvasElement) => { |
|
||||
const canvasWidth = canvasRef.width; |
|
||||
const logoOptions: QrcodeLogo = Object.assign( |
|
||||
{ |
|
||||
logoSize: 0.15, |
|
||||
bgColor: "#ffffff", |
|
||||
borderSize: 0.05, |
|
||||
crossOrigin: "anonymous", |
|
||||
borderRadius: 8, |
|
||||
logoRadius: 0 |
|
||||
}, |
|
||||
isString(props.logo) ? {} : props.logo |
|
||||
); |
|
||||
const { |
|
||||
logoSize = 0.15, |
|
||||
bgColor = "#ffffff", |
|
||||
borderSize = 0.05, |
|
||||
crossOrigin = "anonymous", |
|
||||
borderRadius = 8, |
|
||||
logoRadius = 0 |
|
||||
} = logoOptions; |
|
||||
const logoSrc = isString(props.logo) ? props.logo : props.logo.src; |
|
||||
const logoWidth = canvasWidth * logoSize; |
|
||||
const logoXY = (canvasWidth * (1 - logoSize)) / 2; |
|
||||
const logoBgWidth = canvasWidth * (logoSize + borderSize); |
|
||||
const logoBgXY = (canvasWidth * (1 - logoSize - borderSize)) / 2; |
|
||||
const ctx = canvasRef.getContext("2d"); |
|
||||
if (!ctx) return; |
|
||||
// logo 底色
|
|
||||
canvasRoundRect(ctx)( |
|
||||
logoBgXY, |
|
||||
logoBgXY, |
|
||||
logoBgWidth, |
|
||||
logoBgWidth, |
|
||||
borderRadius |
|
||||
); |
|
||||
ctx.fillStyle = bgColor; |
|
||||
ctx.fill(); |
|
||||
// logo
|
|
||||
const image = new Image(); |
|
||||
if (crossOrigin || logoRadius) { |
|
||||
image.setAttribute("crossOrigin", crossOrigin); |
|
||||
} |
|
||||
(image as any).src = logoSrc; |
|
||||
// 使用image绘制可以避免某些跨域情况
|
|
||||
const drawLogoWithImage = (image: HTMLImageElement) => { |
|
||||
ctx.drawImage(image, logoXY, logoXY, logoWidth, logoWidth); |
|
||||
}; |
|
||||
// 使用canvas绘制以获得更多的功能
|
|
||||
const drawLogoWithCanvas = (image: HTMLImageElement) => { |
|
||||
const canvasImage = document.createElement("canvas"); |
|
||||
canvasImage.width = logoXY + logoWidth; |
|
||||
canvasImage.height = logoXY + logoWidth; |
|
||||
const imageCanvas = canvasImage.getContext("2d"); |
|
||||
if (!imageCanvas || !ctx) return; |
|
||||
imageCanvas.drawImage(image, logoXY, logoXY, logoWidth, logoWidth); |
|
||||
canvasRoundRect(ctx)(logoXY, logoXY, logoWidth, logoWidth, logoRadius); |
|
||||
if (!ctx) return; |
|
||||
const fillStyle = ctx.createPattern(canvasImage, "no-repeat"); |
|
||||
if (fillStyle) { |
|
||||
ctx.fillStyle = fillStyle; |
|
||||
ctx.fill(); |
|
||||
} |
|
||||
}; |
|
||||
// 将 logo绘制到 canvas上
|
|
||||
return new Promise((resolve: any) => { |
|
||||
image.onload = () => { |
|
||||
logoRadius ? drawLogoWithCanvas(image) : drawLogoWithImage(image); |
|
||||
resolve(canvasRef.toDataURL()); |
|
||||
}; |
|
||||
}); |
|
||||
}; |
|
||||
// 得到原QrCode的大小,以便缩放得到正确的QrCode大小
|
|
||||
const getOriginWidth = async ( |
|
||||
content: string, |
|
||||
options: QRCodeRenderersOptions |
|
||||
) => { |
|
||||
const _canvas = document.createElement("canvas"); |
|
||||
await toCanvas(_canvas, content, options); |
|
||||
return _canvas.width; |
|
||||
}; |
|
||||
// 对于内容少的QrCode,增大容错率
|
|
||||
const getErrorCorrectionLevel = (content: string) => { |
|
||||
if (content.length > 36) { |
|
||||
return "M"; |
|
||||
} else if (content.length > 16) { |
|
||||
return "Q"; |
|
||||
} else { |
|
||||
return "H"; |
|
||||
} |
|
||||
}; |
|
||||
// 用于绘制圆角
|
|
||||
const canvasRoundRect = (ctx: CanvasRenderingContext2D) => { |
|
||||
return (x: number, y: number, w: number, h: number, r: number) => { |
|
||||
const minSize = Math.min(w, h); |
|
||||
if (r > minSize / 2) { |
|
||||
r = minSize / 2; |
|
||||
} |
|
||||
ctx.beginPath(); |
|
||||
ctx.moveTo(x + r, y); |
|
||||
ctx.arcTo(x + w, y, x + w, y + h, r); |
|
||||
ctx.arcTo(x + w, y + h, x, y + h, r); |
|
||||
ctx.arcTo(x, y + h, x, y, r); |
|
||||
ctx.arcTo(x, y, x + w, y, r); |
|
||||
ctx.closePath(); |
|
||||
return ctx; |
|
||||
}; |
|
||||
}; |
|
||||
const clickCode = () => { |
|
||||
emit("click"); |
|
||||
}; |
|
||||
const disabledClick = () => { |
|
||||
emit("disabled-click"); |
|
||||
}; |
|
||||
return () => ( |
|
||||
<> |
|
||||
<div |
|
||||
v-loading={unref(loading)} |
|
||||
class="qrcode relative inline-block" |
|
||||
style={unref(wrapStyle)} |
|
||||
> |
|
||||
{props.tag === "canvas" ? ( |
|
||||
<canvas ref={wrapRef} onClick={clickCode}></canvas> |
|
||||
) : ( |
|
||||
<img ref={wrapRef} onClick={clickCode}></img> |
|
||||
)} |
|
||||
{props.disabled && ( |
|
||||
<div |
|
||||
class="qrcode--disabled absolute top-0 left-0 flex w-full h-full items-center justify-center" |
|
||||
onClick={disabledClick} |
|
||||
> |
|
||||
<div class="absolute top-[50%] left-[50%] font-bold"> |
|
||||
<IconifyIconOffline |
|
||||
class="cursor-pointer" |
|
||||
icon="refresh-right" |
|
||||
width="30" |
|
||||
color="var(--el-color-primary)" |
|
||||
/> |
|
||||
<div>{props.disabledText}</div> |
|
||||
</div> |
|
||||
</div> |
|
||||
)} |
|
||||
</div> |
|
||||
</> |
|
||||
); |
|
||||
} |
|
||||
}); |
|
@ -1,106 +0,0 @@ |
|||||
<script setup lang="ts"> |
|
||||
import { useI18n } from "vue-i18n"; |
|
||||
import { ref, reactive } from "vue"; |
|
||||
import Motion from "../utils/motion"; |
|
||||
import { phoneRules } from "../utils/rule"; |
|
||||
import { message } from "@pureadmin/components"; |
|
||||
import type { FormInstance } from "element-plus"; |
|
||||
import { $t, transformI18n } from "/@/plugins/i18n"; |
|
||||
import { useVerifyCode } from "../utils/verifyCode"; |
|
||||
import { useUserStoreHook } from "/@/store/modules/user"; |
|
||||
import { useRenderIcon } from "/@/components/ReIcon/src/hooks"; |
|
||||
|
|
||||
const { t } = useI18n(); |
|
||||
const loading = ref(false); |
|
||||
const ruleForm = reactive({ |
|
||||
phone: "", |
|
||||
verifyCode: "" |
|
||||
}); |
|
||||
const ruleFormRef = ref<FormInstance>(); |
|
||||
const { isDisabled, text } = useVerifyCode(); |
|
||||
|
|
||||
const onLogin = async (formEl: FormInstance | undefined) => { |
|
||||
loading.value = true; |
|
||||
if (!formEl) return; |
|
||||
await formEl.validate((valid, fields) => { |
|
||||
if (valid) { |
|
||||
// 模拟登录请求,需根据实际开发进行修改 |
|
||||
setTimeout(() => { |
|
||||
message.success(transformI18n($t("login.loginSuccess"))); |
|
||||
loading.value = false; |
|
||||
}, 2000); |
|
||||
} else { |
|
||||
loading.value = false; |
|
||||
return fields; |
|
||||
} |
|
||||
}); |
|
||||
}; |
|
||||
|
|
||||
function onBack() { |
|
||||
useVerifyCode().end(); |
|
||||
useUserStoreHook().SET_CURRENTPAGE(0); |
|
||||
} |
|
||||
</script> |
|
||||
|
|
||||
<template> |
|
||||
<el-form ref="ruleFormRef" :model="ruleForm" :rules="phoneRules" size="large"> |
|
||||
<Motion> |
|
||||
<el-form-item prop="phone"> |
|
||||
<el-input |
|
||||
clearable |
|
||||
v-model="ruleForm.phone" |
|
||||
:placeholder="t('login.phone')" |
|
||||
:prefix-icon="useRenderIcon('iphone')" |
|
||||
/> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="100"> |
|
||||
<el-form-item prop="verifyCode"> |
|
||||
<div class="w-full flex justify-between"> |
|
||||
<el-input |
|
||||
clearable |
|
||||
v-model="ruleForm.verifyCode" |
|
||||
:placeholder="t('login.smsVerifyCode')" |
|
||||
:prefix-icon=" |
|
||||
useRenderIcon('ri:shield-keyhole-line', { online: true }) |
|
||||
" |
|
||||
/> |
|
||||
<el-button |
|
||||
:disabled="isDisabled" |
|
||||
class="ml-2" |
|
||||
@click="useVerifyCode().start(ruleFormRef, 'phone')" |
|
||||
> |
|
||||
{{ |
|
||||
text.length > 0 |
|
||||
? text + t("login.info") |
|
||||
: t("login.getVerifyCode") |
|
||||
}} |
|
||||
</el-button> |
|
||||
</div> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="150"> |
|
||||
<el-form-item> |
|
||||
<el-button |
|
||||
class="w-full" |
|
||||
size="default" |
|
||||
type="primary" |
|
||||
:loading="loading" |
|
||||
@click="onLogin(ruleFormRef)" |
|
||||
> |
|
||||
{{ t("login.login") }} |
|
||||
</el-button> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="200"> |
|
||||
<el-form-item> |
|
||||
<el-button class="w-full" size="default" @click="onBack"> |
|
||||
{{ t("login.back") }} |
|
||||
</el-button> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
</el-form> |
|
||||
</template> |
|
@ -1,25 +0,0 @@ |
|||||
<script setup lang="ts"> |
|
||||
import { useI18n } from "vue-i18n"; |
|
||||
import Motion from "../utils/motion"; |
|
||||
import ReQrcode from "/@/components/ReQrcode"; |
|
||||
import { useUserStoreHook } from "/@/store/modules/user"; |
|
||||
|
|
||||
const { t } = useI18n(); |
|
||||
</script> |
|
||||
|
|
||||
<template> |
|
||||
<Motion class="-mt-2 -mb-2"> <ReQrcode :text="t('login.test')" /> </Motion> |
|
||||
<Motion :delay="100"> |
|
||||
<el-divider> |
|
||||
<p class="text-gray-500 text-xs">{{ t("login.tip") }}</p> |
|
||||
</el-divider> |
|
||||
</Motion> |
|
||||
<Motion :delay="150"> |
|
||||
<el-button |
|
||||
class="w-full mt-4" |
|
||||
@click="useUserStoreHook().SET_CURRENTPAGE(0)" |
|
||||
> |
|
||||
{{ t("login.back") }} |
|
||||
</el-button> |
|
||||
</Motion> |
|
||||
</template> |
|
@ -1,189 +0,0 @@ |
|||||
<script setup lang="ts"> |
|
||||
import { useI18n } from "vue-i18n"; |
|
||||
import { ref, reactive } from "vue"; |
|
||||
import Motion from "../utils/motion"; |
|
||||
import { updateRules } from "../utils/rule"; |
|
||||
import { message } from "@pureadmin/components"; |
|
||||
import type { FormInstance } from "element-plus"; |
|
||||
import { useVerifyCode } from "../utils/verifyCode"; |
|
||||
import { $t, transformI18n } from "/@/plugins/i18n"; |
|
||||
import { useUserStoreHook } from "/@/store/modules/user"; |
|
||||
import { useRenderIcon } from "/@/components/ReIcon/src/hooks"; |
|
||||
|
|
||||
const { t } = useI18n(); |
|
||||
const checked = ref(false); |
|
||||
const loading = ref(false); |
|
||||
const ruleForm = reactive({ |
|
||||
username: "", |
|
||||
phone: "", |
|
||||
verifyCode: "", |
|
||||
password: "", |
|
||||
repeatPassword: "" |
|
||||
}); |
|
||||
const ruleFormRef = ref<FormInstance>(); |
|
||||
const { isDisabled, text } = useVerifyCode(); |
|
||||
const repeatPasswordRule = [ |
|
||||
{ |
|
||||
validator: (rule, value, callback) => { |
|
||||
if (value === "") { |
|
||||
callback(new Error(transformI18n($t("login.passwordSureReg")))); |
|
||||
} else if (ruleForm.password !== value) { |
|
||||
callback(new Error(transformI18n($t("login.passwordDifferentReg")))); |
|
||||
} else { |
|
||||
callback(); |
|
||||
} |
|
||||
}, |
|
||||
trigger: "blur" |
|
||||
} |
|
||||
]; |
|
||||
|
|
||||
const onUpdate = async (formEl: FormInstance | undefined) => { |
|
||||
loading.value = true; |
|
||||
if (!formEl) return; |
|
||||
await formEl.validate((valid, fields) => { |
|
||||
if (valid) { |
|
||||
if (checked.value) { |
|
||||
// 模拟请求,需根据实际开发进行修改 |
|
||||
setTimeout(() => { |
|
||||
message.success(transformI18n($t("login.registerSuccess"))); |
|
||||
loading.value = false; |
|
||||
}, 2000); |
|
||||
} else { |
|
||||
loading.value = false; |
|
||||
message.warning(transformI18n($t("login.tickPrivacy"))); |
|
||||
} |
|
||||
} else { |
|
||||
loading.value = false; |
|
||||
return fields; |
|
||||
} |
|
||||
}); |
|
||||
}; |
|
||||
|
|
||||
function onBack() { |
|
||||
useVerifyCode().end(); |
|
||||
useUserStoreHook().SET_CURRENTPAGE(0); |
|
||||
} |
|
||||
</script> |
|
||||
|
|
||||
<template> |
|
||||
<el-form |
|
||||
ref="ruleFormRef" |
|
||||
:model="ruleForm" |
|
||||
:rules="updateRules" |
|
||||
size="large" |
|
||||
> |
|
||||
<Motion> |
|
||||
<el-form-item |
|
||||
:rules="[ |
|
||||
{ |
|
||||
required: true, |
|
||||
message: transformI18n($t('login.usernameReg')), |
|
||||
trigger: 'blur' |
|
||||
} |
|
||||
]" |
|
||||
prop="username" |
|
||||
> |
|
||||
<el-input |
|
||||
clearable |
|
||||
v-model="ruleForm.username" |
|
||||
:placeholder="t('login.username')" |
|
||||
:prefix-icon="useRenderIcon('user')" |
|
||||
/> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="100"> |
|
||||
<el-form-item prop="phone"> |
|
||||
<el-input |
|
||||
clearable |
|
||||
v-model="ruleForm.phone" |
|
||||
:placeholder="t('login.phone')" |
|
||||
:prefix-icon="useRenderIcon('iphone')" |
|
||||
/> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="150"> |
|
||||
<el-form-item prop="verifyCode"> |
|
||||
<div class="w-full flex justify-between"> |
|
||||
<el-input |
|
||||
clearable |
|
||||
v-model="ruleForm.verifyCode" |
|
||||
:placeholder="t('login.smsVerifyCode')" |
|
||||
:prefix-icon=" |
|
||||
useRenderIcon('ri:shield-keyhole-line', { online: true }) |
|
||||
" |
|
||||
/> |
|
||||
<el-button |
|
||||
:disabled="isDisabled" |
|
||||
class="ml-2" |
|
||||
@click="useVerifyCode().start(ruleFormRef, 'phone')" |
|
||||
> |
|
||||
{{ |
|
||||
text.length > 0 |
|
||||
? text + t("login.info") |
|
||||
: t("login.getVerifyCode") |
|
||||
}} |
|
||||
</el-button> |
|
||||
</div> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="200"> |
|
||||
<el-form-item prop="password"> |
|
||||
<el-input |
|
||||
clearable |
|
||||
show-password |
|
||||
v-model="ruleForm.password" |
|
||||
:placeholder="t('login.password')" |
|
||||
:prefix-icon="useRenderIcon('lock')" |
|
||||
/> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="250"> |
|
||||
<el-form-item :rules="repeatPasswordRule" prop="repeatPassword"> |
|
||||
<el-input |
|
||||
clearable |
|
||||
show-password |
|
||||
v-model="ruleForm.repeatPassword" |
|
||||
:placeholder="t('login.sure')" |
|
||||
:prefix-icon="useRenderIcon('lock')" |
|
||||
/> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="300"> |
|
||||
<el-form-item> |
|
||||
<el-checkbox v-model="checked"> |
|
||||
{{ t("login.readAccept") }} |
|
||||
</el-checkbox> |
|
||||
<el-button link type="primary"> |
|
||||
{{ t("login.privacyPolicy") }} |
|
||||
</el-button> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="350"> |
|
||||
<el-form-item> |
|
||||
<el-button |
|
||||
class="w-full" |
|
||||
size="default" |
|
||||
type="primary" |
|
||||
:loading="loading" |
|
||||
@click="onUpdate(ruleFormRef)" |
|
||||
> |
|
||||
{{ t("login.definite") }} |
|
||||
</el-button> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="400"> |
|
||||
<el-form-item> |
|
||||
<el-button class="w-full" size="default" @click="onBack"> |
|
||||
{{ t("login.back") }} |
|
||||
</el-button> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
</el-form> |
|
||||
</template> |
|
@ -1,151 +0,0 @@ |
|||||
<script setup lang="ts"> |
|
||||
import { useI18n } from "vue-i18n"; |
|
||||
import { ref, reactive } from "vue"; |
|
||||
import Motion from "../utils/motion"; |
|
||||
import { updateRules } from "../utils/rule"; |
|
||||
import { message } from "@pureadmin/components"; |
|
||||
import type { FormInstance } from "element-plus"; |
|
||||
import { useVerifyCode } from "../utils/verifyCode"; |
|
||||
import { $t, transformI18n } from "/@/plugins/i18n"; |
|
||||
import { useUserStoreHook } from "/@/store/modules/user"; |
|
||||
import { useRenderIcon } from "/@/components/ReIcon/src/hooks"; |
|
||||
|
|
||||
const { t } = useI18n(); |
|
||||
const loading = ref(false); |
|
||||
const ruleForm = reactive({ |
|
||||
phone: "", |
|
||||
verifyCode: "", |
|
||||
password: "", |
|
||||
repeatPassword: "" |
|
||||
}); |
|
||||
const ruleFormRef = ref<FormInstance>(); |
|
||||
const { isDisabled, text } = useVerifyCode(); |
|
||||
const repeatPasswordRule = [ |
|
||||
{ |
|
||||
validator: (rule, value, callback) => { |
|
||||
if (value === "") { |
|
||||
callback(new Error(transformI18n($t("login.passwordSureReg")))); |
|
||||
} else if (ruleForm.password !== value) { |
|
||||
callback(new Error(transformI18n($t("login.passwordDifferentReg")))); |
|
||||
} else { |
|
||||
callback(); |
|
||||
} |
|
||||
}, |
|
||||
trigger: "blur" |
|
||||
} |
|
||||
]; |
|
||||
|
|
||||
const onUpdate = async (formEl: FormInstance | undefined) => { |
|
||||
loading.value = true; |
|
||||
if (!formEl) return; |
|
||||
await formEl.validate((valid, fields) => { |
|
||||
if (valid) { |
|
||||
// 模拟请求,需根据实际开发进行修改 |
|
||||
setTimeout(() => { |
|
||||
message.success(transformI18n($t("login.passwordUpdateReg"))); |
|
||||
loading.value = false; |
|
||||
}, 2000); |
|
||||
} else { |
|
||||
loading.value = false; |
|
||||
return fields; |
|
||||
} |
|
||||
}); |
|
||||
}; |
|
||||
|
|
||||
function onBack() { |
|
||||
useVerifyCode().end(); |
|
||||
useUserStoreHook().SET_CURRENTPAGE(0); |
|
||||
} |
|
||||
</script> |
|
||||
|
|
||||
<template> |
|
||||
<el-form |
|
||||
ref="ruleFormRef" |
|
||||
:model="ruleForm" |
|
||||
:rules="updateRules" |
|
||||
size="large" |
|
||||
> |
|
||||
<Motion> |
|
||||
<el-form-item prop="phone"> |
|
||||
<el-input |
|
||||
clearable |
|
||||
v-model="ruleForm.phone" |
|
||||
:placeholder="t('login.phone')" |
|
||||
:prefix-icon="useRenderIcon('iphone')" |
|
||||
/> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="100"> |
|
||||
<el-form-item prop="verifyCode"> |
|
||||
<div class="w-full flex justify-between"> |
|
||||
<el-input |
|
||||
clearable |
|
||||
v-model="ruleForm.verifyCode" |
|
||||
:placeholder="t('login.smsVerifyCode')" |
|
||||
:prefix-icon=" |
|
||||
useRenderIcon('ri:shield-keyhole-line', { online: true }) |
|
||||
" |
|
||||
/> |
|
||||
<el-button |
|
||||
:disabled="isDisabled" |
|
||||
class="ml-2" |
|
||||
@click="useVerifyCode().start(ruleFormRef, 'phone')" |
|
||||
> |
|
||||
{{ |
|
||||
text.length > 0 |
|
||||
? text + t("login.info") |
|
||||
: t("login.getVerifyCode") |
|
||||
}} |
|
||||
</el-button> |
|
||||
</div> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="150"> |
|
||||
<el-form-item prop="password"> |
|
||||
<el-input |
|
||||
clearable |
|
||||
show-password |
|
||||
v-model="ruleForm.password" |
|
||||
:placeholder="t('login.password')" |
|
||||
:prefix-icon="useRenderIcon('lock')" |
|
||||
/> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="200"> |
|
||||
<el-form-item :rules="repeatPasswordRule" prop="repeatPassword"> |
|
||||
<el-input |
|
||||
clearable |
|
||||
show-password |
|
||||
v-model="ruleForm.repeatPassword" |
|
||||
:placeholder="t('login.sure')" |
|
||||
:prefix-icon="useRenderIcon('lock')" |
|
||||
/> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="250"> |
|
||||
<el-form-item> |
|
||||
<el-button |
|
||||
class="w-full" |
|
||||
size="default" |
|
||||
type="primary" |
|
||||
:loading="loading" |
|
||||
@click="onUpdate(ruleFormRef)" |
|
||||
> |
|
||||
{{ t("login.definite") }} |
|
||||
</el-button> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
|
|
||||
<Motion :delay="300"> |
|
||||
<el-form-item> |
|
||||
<el-button class="w-full" size="default" @click="onBack"> |
|
||||
{{ t("login.back") }} |
|
||||
</el-button> |
|
||||
</el-form-item> |
|
||||
</Motion> |
|
||||
</el-form> |
|
||||
</template> |
|
@ -1,34 +0,0 @@ |
|||||
import { $t } from "/@/plugins/i18n"; |
|
||||
|
|
||||
const operates = [ |
|
||||
{ |
|
||||
title: $t("login.phoneLogin") |
|
||||
}, |
|
||||
{ |
|
||||
title: $t("login.qRCodeLogin") |
|
||||
}, |
|
||||
{ |
|
||||
title: $t("login.register") |
|
||||
} |
|
||||
]; |
|
||||
|
|
||||
const thirdParty = [ |
|
||||
{ |
|
||||
title: $t("login.weChatLogin"), |
|
||||
icon: "wechat" |
|
||||
}, |
|
||||
{ |
|
||||
title: $t("login.alipayLogin"), |
|
||||
icon: "alipay" |
|
||||
}, |
|
||||
{ |
|
||||
title: $t("login.qqLogin"), |
|
||||
icon: "qq" |
|
||||
}, |
|
||||
{ |
|
||||
title: $t("login.weiboLogin"), |
|
||||
icon: "weibo" |
|
||||
} |
|
||||
]; |
|
||||
|
|
||||
export { operates, thirdParty }; |
|
@ -1,49 +0,0 @@ |
|||||
import type { FormInstance, FormItemProp } from "element-plus"; |
|
||||
import { cloneDeep } from "lodash-unified"; |
|
||||
import { ref } from "vue"; |
|
||||
|
|
||||
const isDisabled = ref(false); |
|
||||
const timer = ref(null); |
|
||||
const text = ref(""); |
|
||||
|
|
||||
export const useVerifyCode = () => { |
|
||||
const start = async ( |
|
||||
formEl: FormInstance | undefined, |
|
||||
props: FormItemProp, |
|
||||
time = 60 |
|
||||
) => { |
|
||||
if (!formEl) return; |
|
||||
const initTime = cloneDeep(time); |
|
||||
await formEl.validateField(props, isValid => { |
|
||||
if (isValid) { |
|
||||
clearInterval(timer.value); |
|
||||
timer.value = setInterval(() => { |
|
||||
if (time > 0) { |
|
||||
text.value = `${time}`; |
|
||||
isDisabled.value = true; |
|
||||
time -= 1; |
|
||||
} else { |
|
||||
text.value = ""; |
|
||||
isDisabled.value = false; |
|
||||
clearInterval(timer.value); |
|
||||
time = initTime; |
|
||||
} |
|
||||
}, 1000); |
|
||||
} |
|
||||
}); |
|
||||
}; |
|
||||
|
|
||||
const end = () => { |
|
||||
text.value = ""; |
|
||||
isDisabled.value = false; |
|
||||
clearInterval(timer.value); |
|
||||
}; |
|
||||
|
|
||||
return { |
|
||||
isDisabled, |
|
||||
timer, |
|
||||
text, |
|
||||
start, |
|
||||
end |
|
||||
}; |
|
||||
}; |
|
Write
Preview
Loading…
Cancel
Save
Reference in new issue