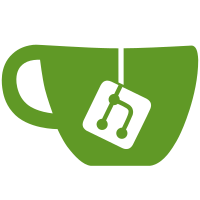
2 changed files with 92 additions and 31 deletions
@ -1,17 +1,39 @@ |
|||||
// 示例使用
|
// 示例使用
|
||||
import { DynamicScriptExecutor } from '@/utils/dynamic_script_executor.ts' |
import { DynamicScriptExecutor } from '@/utils/dynamic_script_executor.ts' |
||||
|
|
||||
const executor = new DynamicScriptExecutor({ |
|
||||
|
// 示例使用
|
||||
|
const inputString = `
|
||||
|
This is some text. |
||||
|
{{ console.log("Dynamic JS block 1"); }} |
||||
|
More text here. |
||||
|
<% |
||||
|
let x = 10; |
||||
|
let y = 20; |
||||
|
console.log("Dynamic JS block 2: ", x + y); |
||||
|
%> |
||||
|
And some final text. |
||||
|
`
|
||||
|
|
||||
|
// 使用默认的 {{ 和 }} 标记
|
||||
|
const executorDefault = new DynamicScriptExecutor({ |
||||
user: { name: 'Alice', age: 30 }, |
user: { name: 'Alice', age: 30 }, |
||||
sayHello: (name: string) => `Hello, ${name}!` |
sayHello: (name: string) => `Hello, ${name}!` |
||||
}) |
}) |
||||
|
|
||||
|
// 使用默认标记执行动态JS块
|
||||
|
executorDefault.execute(inputString) |
||||
|
.then(results => console.log('执行结果(默认标记):', results)) |
||||
|
.catch(error => console.error('捕获到的错误:', error)) |
||||
|
|
||||
|
// 使用自定义的 <% 和 %> 标记
|
||||
|
const executorCustom = new DynamicScriptExecutor( |
||||
|
{ user: { name: 'Alice', age: 30 }, sayHello: (name: string) => `Hello, ${name}!` }, |
||||
|
'<%', |
||||
|
'%>' |
||||
|
) |
||||
|
|
||||
// 代码块中有可能抛出异常的代码
|
|
||||
const result = executor.execute(`
|
|
||||
console.log(user); |
|
||||
throw new Error('测试错误'); // 故意抛出一个错误
|
|
||||
return sayHello(user.name); |
|
||||
`)
|
|
||||
|
// 使用自定义标记执行动态JS块
|
||||
|
executorCustom.execute(inputString) |
||||
|
.then(results => console.log('执行结果(自定义标记):', results)) |
||||
|
.catch(error => console.error('捕获到的错误:', error)) |
||||
|
|
||||
result.then(console.log).catch(console.error) |
|
Write
Preview
Loading…
Cancel
Save
Reference in new issue