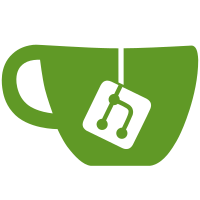
19 changed files with 815 additions and 569 deletions
-
95src/components/table/Table.tsx
-
1src/components/table/index.ts
-
23src/components/table/style.ts
-
4src/hooks/useScrollStyle.ts
-
3src/layout/ListPageLayout.tsx
-
5src/layout/TwoColPageLayout.tsx
-
36src/layout/style.ts
-
12src/pages/cms/collect/index.tsx
-
12src/pages/cms/video/index.tsx
-
12src/pages/cms/video_cloud/index.tsx
-
12src/pages/cms/video_magnet/index.tsx
-
32src/pages/system/logs/login/index.tsx
-
4src/pages/system/menus/index.tsx
-
13src/pages/system/menus/style.ts
-
7src/pages/system/roles/index.tsx
-
7src/pages/system/users/index.tsx
-
9src/pages/videos/list/index.tsx
-
11src/pages/websites/ssl/index.tsx
-
12src/utils/dom.ts
@ -0,0 +1,95 @@ |
|||||
|
import { ProTable, ProTableProps, ProCard } from '@ant-design/pro-components' |
||||
|
import React, { useEffect, useRef, useState } from 'react' |
||||
|
import { useStyle } from './style' |
||||
|
|
||||
|
export interface TableProps<T = any, D = any> extends ProTableProps<T, D> { |
||||
|
|
||||
|
} |
||||
|
|
||||
|
export const Table = <T extends Record<string, any> = any, D = any>(props: TableProps<T, D>) => { |
||||
|
|
||||
|
const { styles } = useStyle() |
||||
|
const toolbarRef = useRef<HTMLDivElement | undefined | null>(undefined) |
||||
|
const alterRef = useRef<HTMLDivElement | undefined | null>(undefined) |
||||
|
const [ toolbarHeight, setHeight ] = useState<number>(65) |
||||
|
const [ alterHeight, setAlterHeight ] = useState<number>(0) |
||||
|
|
||||
|
const scroll = props.scroll ? { |
||||
|
...props.scroll, |
||||
|
y: props.scroll.y ?? ` calc(100vh - ${toolbarHeight + 200}px)` |
||||
|
} : undefined |
||||
|
|
||||
|
useEffect(() => { |
||||
|
|
||||
|
if (!toolbarRef.current) return |
||||
|
|
||||
|
setHeight(toolbarRef.current?.offsetHeight ?? 65) |
||||
|
|
||||
|
//监听toolbarRef offsetHeight
|
||||
|
const observer = new ResizeObserver(entries => { |
||||
|
for (const entry of entries) { |
||||
|
if (entry.target === toolbarRef.current) { |
||||
|
setHeight(entry.contentRect.height) |
||||
|
} |
||||
|
} |
||||
|
}) |
||||
|
|
||||
|
observer.observe(toolbarRef.current!) |
||||
|
|
||||
|
return () => { |
||||
|
observer.disconnect() |
||||
|
} |
||||
|
|
||||
|
}, [ toolbarRef.current ]) |
||||
|
|
||||
|
useEffect(() => { |
||||
|
|
||||
|
if (!alterRef.current) return |
||||
|
|
||||
|
setHeight(alterRef.current?.offsetHeight ?? 65) |
||||
|
|
||||
|
//监听toolbarRef offsetHeight
|
||||
|
const observer = new ResizeObserver(entries => { |
||||
|
for (const entry of entries) { |
||||
|
if (entry.target === alterRef.current && entry.contentRect.height > 0) { |
||||
|
setAlterHeight(entry.contentRect.height + 16) |
||||
|
} |
||||
|
} |
||||
|
}) |
||||
|
|
||||
|
observer.observe(alterRef.current!) |
||||
|
|
||||
|
return () => { |
||||
|
observer.disconnect() |
||||
|
} |
||||
|
|
||||
|
}, [ alterRef.current ]) |
||||
|
|
||||
|
const style = { |
||||
|
'--toolbar-height': `${toolbarHeight}px`, |
||||
|
'--alter-height': `${alterHeight}px`, |
||||
|
} as React.CSSProperties |
||||
|
|
||||
|
// @ts-ignore fix dataItem
|
||||
|
return <ProTable<T> |
||||
|
{...props} |
||||
|
className={styles.container} |
||||
|
style={style} |
||||
|
tableRender={(props, _dom, domList) => { |
||||
|
return <ProCard |
||||
|
ghost={props.ghost} |
||||
|
{...props.cardProps} |
||||
|
bodyStyle={{ |
||||
|
paddingBlockStart: 0, |
||||
|
}} |
||||
|
> |
||||
|
<div ref={toolbarRef as any}>{domList.toolbar}</div> |
||||
|
<div ref={alterRef as any}>{domList.alert}</div> |
||||
|
<>{domList.table}</> |
||||
|
</ProCard> |
||||
|
}} |
||||
|
scroll={scroll} |
||||
|
></ProTable> |
||||
|
} |
||||
|
|
||||
|
export default Table |
@ -0,0 +1 @@ |
|||||
|
export * from './Table.tsx' |
@ -0,0 +1,23 @@ |
|||||
|
import { createStyles } from '@/theme' |
||||
|
|
||||
|
export const useStyle = createStyles(({ token, css, cx, prefixCls }, props: any) => { |
||||
|
const prefix = `${prefixCls}-${token?.proPrefix}-my-table` |
||||
|
|
||||
|
const container = css`
|
||||
|
|
||||
|
--toolbar-height: 65px; |
||||
|
--alter-height: 0px; |
||||
|
--padding: 37px; |
||||
|
--table-body-height: calc(var(--toolbar-height, 65px) + var(--alter-height, 0px) + var(--header-height, 56px) + var(--padding, 20px) * 4); |
||||
|
|
||||
|
.ant-table-body { |
||||
|
overflow: auto scroll; |
||||
|
max-height: calc(100vh - var(--table-body-height)) !important; |
||||
|
height: calc(100vh - var(--table-body-height)) !important; |
||||
|
} |
||||
|
`
|
||||
|
|
||||
|
return { |
||||
|
container: cx(prefix, props?.className, container), |
||||
|
} |
||||
|
}) |
@ -0,0 +1,12 @@ |
|||||
|
export function getElementRealHeight(el: Element) { |
||||
|
const style = window.getComputedStyle(el) |
||||
|
|
||||
|
const paddingTop = parseInt(style.paddingTop) |
||||
|
const paddingBottom = parseInt(style.paddingBottom) |
||||
|
const marginTop = parseInt(style.marginTop) |
||||
|
const marginBottom = parseInt(style.marginBottom) |
||||
|
|
||||
|
const clientHeight = el.clientHeight |
||||
|
|
||||
|
return clientHeight + paddingTop + paddingBottom + marginTop + marginBottom |
||||
|
} |
Write
Preview
Loading…
Cancel
Save
Reference in new issue