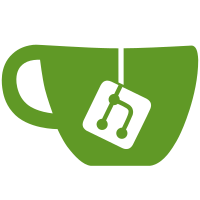
4 changed files with 153 additions and 35 deletions
-
10src/components/dialog/useDialog.tsx
-
37src/components/modal-pro/index.tsx
-
21src/components/table/Table.tsx
-
118src/components/table/style.ts
@ -0,0 +1,37 @@ |
|||||
|
import { Modal, ModalFuncProps } from 'antd' |
||||
|
import React from 'react' |
||||
|
import { HookAPI } from 'antd/es/modal/useModal' |
||||
|
import { useDialog } from '@/components/dialog' |
||||
|
|
||||
|
export interface AlterProps extends ModalFuncProps { |
||||
|
onLoad?: () => void |
||||
|
alterType: keyof HookAPI | 'dialog' |
||||
|
children: JSX.Element | React.ReactNode |
||||
|
|
||||
|
} |
||||
|
|
||||
|
const ModalPro = ({ onLoad, alterType, children, ...props }: AlterProps) => { |
||||
|
|
||||
|
const [ modal, modalHolder ] = Modal.useModal() |
||||
|
const [ dialogRef, dialog, ] = useDialog(props) |
||||
|
|
||||
|
|
||||
|
return ( |
||||
|
<> |
||||
|
<span onClick={() => { |
||||
|
if (onLoad) { |
||||
|
onLoad() |
||||
|
} |
||||
|
if (alterType === 'dialog') { |
||||
|
dialogRef.current?.show() |
||||
|
} else { |
||||
|
modal[alterType]?.(props) |
||||
|
} |
||||
|
}}>{children}</span> |
||||
|
{alterType !== 'dialog' && modalHolder} |
||||
|
{alterType === 'dialog' && dialog} |
||||
|
</> |
||||
|
) |
||||
|
} |
||||
|
|
||||
|
export default ModalPro |
@ -1,48 +1,106 @@ |
|||||
import { createStyles } from '@/theme' |
import { createStyles } from '@/theme' |
||||
import { useScrollStyle } from '@/hooks/useScrollStyle.ts' |
import { useScrollStyle } from '@/hooks/useScrollStyle.ts' |
||||
|
import { unit } from '@ant-design/cssinjs' |
||||
|
|
||||
export const useStyle = createStyles(({ token, css, cx, prefixCls }, props: any) => { |
export const useStyle = createStyles(({ token, css, cx, prefixCls }, props: any) => { |
||||
const prefix = `${prefixCls}-${token?.proPrefix}-my-table` |
|
||||
const { scrollbar } = useScrollStyle() |
|
||||
|
const prefix = `${prefixCls}-${token?.proPrefix}-my-table` |
||||
|
const { scrollbar } = useScrollStyle() |
||||
|
|
||||
const container = css`
|
|
||||
|
const container = css`
|
||||
|
|
||||
--toolbar-height: 65px; |
|
||||
--alter-height: 0px; |
|
||||
--padding: 33px; |
|
||||
--table-body-height: calc( var(--pageHeader, 0px) + var(--toolbar-height, 65px) + var(--alter-height, 0px) + var(--header-height, 56px) + var(--padding, 20px) * 4); |
|
||||
|
--toolbar-height: 65px; |
||||
|
--alter-height: 0px; |
||||
|
--padding: 33px; |
||||
|
--table-body-height: calc(var(--pageHeader, 0px) + var(--toolbar-height, 65px) + var(--alter-height, 0px) + var(--header-height, 56px) + var(--padding, 20px) * 4); |
||||
|
|
||||
.ant-table-body { |
|
||||
overflow: auto scroll; |
|
||||
max-height: calc(100vh - var(--table-body-height)) !important; |
|
||||
height: calc(100vh - var(--table-body-height)) !important; |
|
||||
|
.ant-table-body { |
||||
|
overflow: auto scroll; |
||||
|
max-height: calc(100vh - var(--table-body-height)) !important; |
||||
|
height: calc(100vh - var(--table-body-height)) !important; |
||||
|
|
||||
} |
|
||||
|
} |
||||
|
|
||||
|
.ant-table-wrapper .ant-table { |
||||
|
scrollbar-color: unset; |
||||
|
} |
||||
|
|
||||
.ant-table-wrapper .ant-table{ |
|
||||
scrollbar-color: unset; |
|
||||
|
.ant-table-wrapper { |
||||
|
.ant-pagination { |
||||
|
display: none; |
||||
} |
} |
||||
.ant-table-body{ |
|
||||
|
} |
||||
|
|
||||
${scrollbar} |
|
||||
|
.ant-table-body { |
||||
|
|
||||
} |
|
||||
.ant-table-pagination.ant-pagination { |
|
||||
border-top: 1px solid #ebeef5; |
|
||||
height: 51px; |
|
||||
margin: 0; |
|
||||
align-content: center; |
|
||||
} |
|
||||
|
${scrollbar} |
||||
|
|
||||
.ant-table-cell{ |
|
||||
.ant-divider-vertical{ |
|
||||
margin-inline: 0; |
|
||||
} |
|
||||
|
} |
||||
|
|
||||
|
.ant-table-pagination.ant-pagination { |
||||
|
margin: ${unit(token.margin)} 0; |
||||
|
} |
||||
|
|
||||
|
|
||||
|
.ant-table-cell { |
||||
|
.ant-divider-vertical { |
||||
|
margin-inline: 0; |
||||
} |
} |
||||
`
|
|
||||
|
} |
||||
|
`
|
||||
|
|
||||
return { |
|
||||
container: cx(prefix, props?.className, container), |
|
||||
|
const pagination = { |
||||
|
|
||||
|
borderTop: '1px solid #ebeef5', |
||||
|
height: '51px', |
||||
|
margin: '0', |
||||
|
alignContent: 'center', |
||||
|
display: 'flex', |
||||
|
flexWrap: 'wrap', |
||||
|
rowGap: token.paddingXS, |
||||
|
justifyContent: 'flex-end', |
||||
|
|
||||
|
|
||||
|
'> *': { |
||||
|
flex: 'none', |
||||
|
}, |
||||
|
|
||||
|
'&-left': { |
||||
|
justifyContent: 'flex-start', |
||||
|
}, |
||||
|
|
||||
|
'&-center': { |
||||
|
justifyContent: 'center', |
||||
|
}, |
||||
|
|
||||
|
'&-right': { |
||||
|
justifyContent: 'flex-end', |
||||
|
}, |
||||
|
} |
||||
|
|
||||
|
const footer = css`
|
||||
|
display: flex; |
||||
|
justify-content: space-between; |
||||
|
align-items: center; |
||||
|
overflow: hidden; |
||||
|
|
||||
|
.ant-pro-table-alert{ |
||||
|
max-width: 50%; |
||||
|
overflow: auto; |
||||
|
margin-block-end: 0; |
||||
|
background-color: transparent; |
||||
|
|
||||
|
.ant-pro-table-alert-container{ |
||||
|
padding-inline: 0; |
||||
|
} |
||||
|
} |
||||
|
&-fill{ |
||||
|
flex: 1; |
||||
} |
} |
||||
|
`
|
||||
|
|
||||
|
return { |
||||
|
container: cx(prefix, props?.className, container), |
||||
|
pagination: pagination as any, |
||||
|
footer, |
||||
|
} |
||||
}) |
}) |
Write
Preview
Loading…
Cancel
Save
Reference in new issue