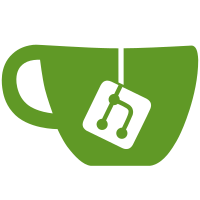
31 changed files with 337 additions and 308 deletions
-
2src/App.tsx
-
2src/Auth.tsx
-
2src/context.ts
-
0src/global.d.ts
-
2src/hooks/useFetch.ts
-
2src/i18n.ts
-
2src/layout/RootLayout.tsx
-
8src/pages/system/departments/components/DepartmentTree.tsx
-
8src/pages/system/departments/components/TreeNodeRender.tsx
-
255src/pages/system/departments/index.tsx
-
6src/pages/system/menus/components/BatchButton.tsx
-
2src/pages/system/menus/components/ButtonTable.tsx
-
10src/pages/system/menus/components/MenuTree.tsx
-
8src/pages/system/menus/components/TreeNodeRender.tsx
-
10src/pages/system/menus/index.tsx
-
16src/pages/system/roles/index.tsx
-
2src/request.ts
-
2src/routes.tsx
-
2src/service/base.ts
-
8src/service/system.ts
-
2src/store/department.ts
-
2src/store/menu.ts
-
2src/store/role.ts
-
2src/store/route.ts
-
2src/store/system.ts
-
86src/store/user.ts
-
5src/types/index.ts
-
3src/types/menus.d.ts
-
2src/utils/index.ts
-
2src/utils/uuid.ts
@ -1,59 +1,77 @@ |
|||||
import { appAtom } from '@/store/system.ts' |
import { appAtom } from '@/store/system.ts' |
||||
import { IMenu } from '@/types/menus' |
import { IMenu } from '@/types/menus' |
||||
import { IUserInfo } from '@/types/user' |
|
||||
|
import { IUserInfo } from '@/types/user' |
||||
import { atom } from 'jotai/index' |
import { atom } from 'jotai/index' |
||||
import { IApiResult, IAuth, IPageResult, MenuItem } from '@/types' |
|
||||
|
import { IApiResult, IAuth, IPageResult, MenuItem } from '@/global' |
||||
import { LoginRequest } from '@/types/login' |
import { LoginRequest } from '@/types/login' |
||||
import { atomWithMutation, atomWithQuery } from 'jotai-tanstack-query' |
import { atomWithMutation, atomWithQuery } from 'jotai-tanstack-query' |
||||
import systemServ from '@/service/system.ts' |
import systemServ from '@/service/system.ts' |
||||
import { formatMenuData, isDev } from '@/utils' |
import { formatMenuData, isDev } from '@/utils' |
||||
|
|
||||
export const authAtom = atom<IAuth>({ |
export const authAtom = atom<IAuth>({ |
||||
isLogin: false, |
|
||||
authKey: [] |
|
||||
|
isLogin: false, |
||||
|
authKey: [] |
||||
}) |
}) |
||||
|
|
||||
const devLogin = { |
const devLogin = { |
||||
username: 'SupperAdmin', |
|
||||
password: 'kk123456', |
|
||||
code: '123456' |
|
||||
|
username: 'SupperAdmin', |
||||
|
password: 'kk123456', |
||||
|
code: '123456' |
||||
} |
} |
||||
export const loginFormAtom = atom<LoginRequest>({ |
export const loginFormAtom = atom<LoginRequest>({ |
||||
...(isDev ? devLogin : {}) |
|
||||
|
...(isDev ? devLogin : {}) |
||||
} as LoginRequest) |
} as LoginRequest) |
||||
|
|
||||
export const loginAtom = atomWithMutation<any, LoginRequest>(() => ({ |
export const loginAtom = atomWithMutation<any, LoginRequest>(() => ({ |
||||
mutationKey: [ 'login' ], |
|
||||
mutationFn: async (params) => { |
|
||||
return await systemServ.login(params) |
|
||||
}, |
|
||||
onSuccess: () => { |
|
||||
// console.log('login success', data)
|
|
||||
}, |
|
||||
retry: false, |
|
||||
|
mutationKey: [ 'login' ], |
||||
|
mutationFn: async (params) => { |
||||
|
return await systemServ.login(params) |
||||
|
}, |
||||
|
onSuccess: () => { |
||||
|
// console.log('login success', data)
|
||||
|
}, |
||||
|
retry: false, |
||||
})) |
})) |
||||
|
|
||||
export const currentUserAtom = atomWithQuery<IApiResult<IUserInfo>, any, IUserInfo>((get) => { |
export const currentUserAtom = atomWithQuery<IApiResult<IUserInfo>, any, IUserInfo>((get) => { |
||||
return { |
|
||||
queryKey: [ 'user_info', get(appAtom).token ], |
|
||||
queryFn: async () => { |
|
||||
return await systemServ.user.current() |
|
||||
}, |
|
||||
select: (data) => { |
|
||||
return data.data |
|
||||
|
return { |
||||
|
queryKey: [ 'user_info', get(appAtom).token ], |
||||
|
queryFn: async () => { |
||||
|
return await systemServ.user.current() |
||||
|
}, |
||||
|
select: (data) => { |
||||
|
return data.data |
||||
|
} |
||||
} |
} |
||||
} |
|
||||
}) |
}) |
||||
|
|
||||
export const userMenuDataAtom = atomWithQuery<IApiResult<IPageResult<IMenu[]>>, any, MenuItem[]>((get) => ({ |
export const userMenuDataAtom = atomWithQuery<IApiResult<IPageResult<IMenu[]>>, any, MenuItem[]>((get) => ({ |
||||
enabled: false, |
|
||||
queryKey: [ 'user_menus', get(appAtom).token ], |
|
||||
queryFn: async () => { |
|
||||
return await systemServ.user.menus() |
|
||||
}, |
|
||||
select: (data) => { |
|
||||
return formatMenuData(data.data.rows as any ?? []) |
|
||||
}, |
|
||||
cacheTime: 1000 * 60, |
|
||||
retry: false, |
|
||||
|
enabled: false, |
||||
|
queryKey: [ 'user_menus', get(appAtom).token ], |
||||
|
queryFn: async () => { |
||||
|
return await systemServ.user.menus() |
||||
|
}, |
||||
|
select: (data) => { |
||||
|
return formatMenuData(data.data.rows as any ?? []) |
||||
|
}, |
||||
|
cacheTime: 1000 * 60, |
||||
|
retry: false, |
||||
})) |
})) |
||||
|
|
||||
|
export const userSearchAtom = atom<{ |
||||
|
dept_id: number, |
||||
|
key: string |
||||
|
} | unknown>({}) |
||||
|
|
||||
|
//user list
|
||||
|
export const userListAtom = atomWithQuery((get) => { |
||||
|
return { |
||||
|
queryKey: [ 'user_list', get(userSearchAtom) ], |
||||
|
queryFn: async ({ queryKey: [ , params ] }) => { |
||||
|
return await systemServ.user.list(params) |
||||
|
}, |
||||
|
select: (data) => { |
||||
|
return data.data |
||||
|
}, |
||||
|
} |
||||
|
}) |
@ -0,0 +1,5 @@ |
|||||
|
export * from './department' |
||||
|
export * from './user' |
||||
|
export * from './login' |
||||
|
export * from './roles' |
||||
|
export * from './menus' |
Write
Preview
Loading…
Cancel
Save
Reference in new issue