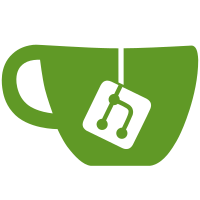
11 changed files with 349 additions and 19 deletions
-
8src/App.css
-
95src/components/dialog/index.tsx
-
33src/components/dialog/style.ts
-
26src/components/dialog/useDialog.tsx
-
51src/components/icon/index.tsx
-
5src/layout/RootLayout.tsx
-
21src/locales/lang/pages/websites/zh-CN.ts
-
7src/locales/lang/zh-CN.ts
-
2src/pages/websites/dns/DNSList.tsx
-
80src/pages/websites/domain/index.tsx
-
32src/store/websites/dns_account.ts
@ -0,0 +1,95 @@ |
|||||
|
import { Modal, ModalProps } from 'antd' |
||||
|
import { useStyle } from './style' |
||||
|
import { |
||||
|
useState, |
||||
|
forwardRef, |
||||
|
ReactNode, |
||||
|
useImperativeHandle, |
||||
|
useCallback, useRef |
||||
|
} from 'react' |
||||
|
|
||||
|
export { useDialog } from './useDialog' |
||||
|
|
||||
|
export interface DialogRef { |
||||
|
show: (data?: any) => void |
||||
|
close: () => void |
||||
|
|
||||
|
get data() |
||||
|
} |
||||
|
|
||||
|
export interface DialogProps extends Omit<ModalProps, 'onCancel' | 'onOk'> { |
||||
|
target?: ReactNode | JSX.Element |
||||
|
ref?: DialogRef |
||||
|
onCancel?: () => boolean | void |
||||
|
onOk?: (data?: any) => Promise<boolean | void> | boolean | void |
||||
|
} |
||||
|
|
||||
|
const Dialog = forwardRef<DialogRef, DialogProps>(({ open, destroyOnClose, target, ...props }: DialogProps, ref) => { |
||||
|
|
||||
|
const { styles, cx } = useStyle() |
||||
|
const [ innerOpen, setOpen ] = useState(() => open ?? false) |
||||
|
const [ submitting, setSubmitting ] = useState(false) |
||||
|
|
||||
|
const dataRef = useRef<any>() |
||||
|
|
||||
|
useImperativeHandle(ref, () => { |
||||
|
return { |
||||
|
show: (data?: any) => { |
||||
|
dataRef.current = data |
||||
|
setOpen(true) |
||||
|
}, |
||||
|
close: () => { |
||||
|
setOpen(false) |
||||
|
}, |
||||
|
get data() { |
||||
|
return dataRef.current |
||||
|
} |
||||
|
} |
||||
|
}, [ setOpen ]) |
||||
|
|
||||
|
const renderTarget = useCallback(() => { |
||||
|
if (target) { |
||||
|
return <span onClick={() => { |
||||
|
setOpen(true) |
||||
|
}}> |
||||
|
{target} |
||||
|
</span> |
||||
|
} |
||||
|
return null |
||||
|
|
||||
|
}, [ target ]) |
||||
|
|
||||
|
return ( |
||||
|
<div className={styles.container}> |
||||
|
{renderTarget()} |
||||
|
<Modal |
||||
|
destroyOnClose={destroyOnClose ?? true} |
||||
|
{...props} |
||||
|
wrapClassName={cx(styles.wrap, props.wrapClassName)} |
||||
|
confirmLoading={submitting} |
||||
|
open={innerOpen} |
||||
|
onCancel={() => { |
||||
|
if (props.onCancel?.() === false) { |
||||
|
return |
||||
|
} |
||||
|
setOpen(false) |
||||
|
}} |
||||
|
onOk={async () => { |
||||
|
if (props.onOk) { |
||||
|
setSubmitting(true) |
||||
|
const res = await props.onOk(dataRef.current) |
||||
|
setSubmitting(false) |
||||
|
if (res === false) { |
||||
|
return |
||||
|
} |
||||
|
} |
||||
|
setOpen(false) |
||||
|
}} |
||||
|
> |
||||
|
{props.children} |
||||
|
</Modal> |
||||
|
</div> |
||||
|
) |
||||
|
}) |
||||
|
|
||||
|
export default Dialog |
@ -0,0 +1,33 @@ |
|||||
|
import { createStyles } from '@/theme' |
||||
|
|
||||
|
export const useStyle = createStyles(({ token, css, cx, prefixCls }, props: any) => { |
||||
|
const prefix = `${prefixCls}-${token?.proPrefix}-dialog-component` |
||||
|
|
||||
|
const container = css`
|
||||
|
|
||||
|
`
|
||||
|
|
||||
|
const wrap = css`
|
||||
|
.ant-modal-content{ |
||||
|
padding: 20px 0 15px 0; |
||||
|
|
||||
|
.ant-modal-header{ |
||||
|
padding: 0 24px 10px; |
||||
|
border-bottom: 1px solid #eee; |
||||
|
} |
||||
|
.ant-modal-body{ |
||||
|
padding: 10px 24px; |
||||
|
} |
||||
|
.ant-modal-footer{ |
||||
|
background-color: #fcfcfc; |
||||
|
padding: 15px 24px 0; |
||||
|
border-top: 1px solid #eee; |
||||
|
} |
||||
|
} |
||||
|
`
|
||||
|
|
||||
|
return { |
||||
|
container: cx(prefix, props?.className, container), |
||||
|
wrap, |
||||
|
} |
||||
|
}) |
@ -0,0 +1,26 @@ |
|||||
|
import Dialog, { DialogProps, DialogRef } from './index.tsx' |
||||
|
import { useRef, useCallback, useMemo } from 'react' |
||||
|
|
||||
|
export const useDialog = (props: DialogProps) => { |
||||
|
const dialogRef = useRef<DialogRef | undefined>() |
||||
|
|
||||
|
const openDialog = useCallback((data?: any) => { |
||||
|
if (dialogRef.current) { |
||||
|
dialogRef.current.show(data) |
||||
|
} else { |
||||
|
console.error('Dialog ref is not defined') |
||||
|
} |
||||
|
}, []) |
||||
|
|
||||
|
const closeDialog = useCallback(() => { |
||||
|
if (dialogRef.current) { |
||||
|
dialogRef.current.close() |
||||
|
} else { |
||||
|
console.error('Dialog ref is not defined') |
||||
|
} |
||||
|
}, []) |
||||
|
|
||||
|
const dialog = useMemo(() => <Dialog {...props} ref={dialogRef as any}/>, [ props ]) |
||||
|
|
||||
|
return [ dialogRef, dialog, openDialog, closeDialog ] as const |
||||
|
} |
@ -0,0 +1,21 @@ |
|||||
|
export default { |
||||
|
status: { |
||||
|
/** |
||||
|
* StatusSuccess StatusEnum = "success" // 成功
|
||||
|
* StatusFail StatusEnum = "fail" // 失败
|
||||
|
* StatusEnable StatusEnum = "enable" // 启用
|
||||
|
* StatusPending StatusEnum = "pending" // 等待
|
||||
|
* StatusDelete StatusEnum = "delete" // 删除
|
||||
|
* StatusDisable StatusEnum = "disable" // 禁用
|
||||
|
* StatusSyncing StatusEnum = "syncing" // 同步
|
||||
|
*/ |
||||
|
success: '成功', |
||||
|
fail: '失败', |
||||
|
enable: '启用', |
||||
|
pending: '等待', |
||||
|
delete: '删除', |
||||
|
disable: '禁用', |
||||
|
syncing: '同步', |
||||
|
|
||||
|
} |
||||
|
} |
Write
Preview
Loading…
Cancel
Save
Reference in new issue