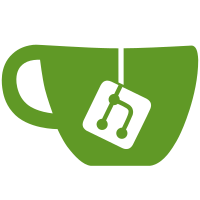
28 changed files with 1644 additions and 431 deletions
-
2build/plugins.ts
-
8package.json
-
392pnpm-lock.yaml
-
7src/components/ReIcon/src/iconifyIconOnline.ts
-
12src/components/ReImageVerify/index.ts
-
85src/components/ReImageVerify/src/hooks.ts
-
48src/components/ReImageVerify/src/index.vue
-
10src/components/ReQrcode/index.ts
-
8src/components/ReQrcode/src/index.scss
-
262src/components/ReQrcode/src/index.tsx
-
2src/router/modules/remaining.ts
-
2src/store/modules/types.ts
-
12src/store/modules/user.ts
-
141src/style/login.css
-
10src/style/sidebar.scss
-
22src/utils/is.ts
-
4src/utils/useComponent.ts
-
152src/views/login.vue
-
96src/views/login/components/phone.vue
-
22src/views/login/components/qrCode.vue
-
169src/views/login/components/regist.vue
-
141src/views/login/components/update.vue
-
208src/views/login/index.vue
-
32src/views/login/utils/enums.ts
-
40src/views/login/utils/motion.ts
-
128src/views/login/utils/rule.ts
-
10src/views/login/utils/static.ts
-
50src/views/login/utils/verifyCode.ts
@ -0,0 +1,12 @@ |
|||
import { App } from "vue"; |
|||
import reImageVerify from "./src/index.vue"; |
|||
|
|||
export const ReImageVerify = Object.assign(reImageVerify, { |
|||
install(app: App) { |
|||
app.component(reImageVerify.name, reImageVerify); |
|||
} |
|||
}); |
|||
|
|||
export default { |
|||
ReImageVerify |
|||
}; |
@ -0,0 +1,85 @@ |
|||
import { ref, onMounted } from "vue"; |
|||
|
|||
/** |
|||
* 绘制图形验证码 |
|||
* @param width - 图形宽度 |
|||
* @param height - 图形高度 |
|||
*/ |
|||
export const useImageVerify = (width = 120, height = 40) => { |
|||
const domRef = ref<HTMLCanvasElement>(); |
|||
const imgCode = ref(""); |
|||
|
|||
function setImgCode(code: string) { |
|||
imgCode.value = code; |
|||
} |
|||
|
|||
function getImgCode() { |
|||
if (!domRef.value) return; |
|||
imgCode.value = draw(domRef.value, width, height); |
|||
} |
|||
|
|||
onMounted(() => { |
|||
getImgCode(); |
|||
}); |
|||
|
|||
return { |
|||
domRef, |
|||
imgCode, |
|||
setImgCode, |
|||
getImgCode |
|||
}; |
|||
}; |
|||
|
|||
function randomNum(min: number, max: number) { |
|||
const num = Math.floor(Math.random() * (max - min) + min); |
|||
return num; |
|||
} |
|||
|
|||
function randomColor(min: number, max: number) { |
|||
const r = randomNum(min, max); |
|||
const g = randomNum(min, max); |
|||
const b = randomNum(min, max); |
|||
return `rgb(${r},${g},${b})`; |
|||
} |
|||
|
|||
function draw(dom: HTMLCanvasElement, width: number, height: number) { |
|||
let imgCode = ""; |
|||
|
|||
const NUMBER_STRING = "0123456789"; |
|||
|
|||
const ctx = dom.getContext("2d"); |
|||
if (!ctx) return imgCode; |
|||
|
|||
ctx.fillStyle = randomColor(180, 230); |
|||
ctx.fillRect(0, 0, width, height); |
|||
for (let i = 0; i < 4; i += 1) { |
|||
const text = NUMBER_STRING[randomNum(0, NUMBER_STRING.length)]; |
|||
imgCode += text; |
|||
const fontSize = randomNum(18, 41); |
|||
const deg = randomNum(-30, 30); |
|||
ctx.font = `${fontSize}px Simhei`; |
|||
ctx.textBaseline = "top"; |
|||
ctx.fillStyle = randomColor(80, 150); |
|||
ctx.save(); |
|||
ctx.translate(30 * i + 15, 15); |
|||
ctx.rotate((deg * Math.PI) / 180); |
|||
ctx.fillText(text, -15 + 5, -15); |
|||
ctx.restore(); |
|||
} |
|||
for (let i = 0; i < 5; i += 1) { |
|||
ctx.beginPath(); |
|||
ctx.moveTo(randomNum(0, width), randomNum(0, height)); |
|||
ctx.lineTo(randomNum(0, width), randomNum(0, height)); |
|||
ctx.strokeStyle = randomColor(180, 230); |
|||
ctx.closePath(); |
|||
ctx.stroke(); |
|||
} |
|||
for (let i = 0; i < 41; i += 1) { |
|||
ctx.beginPath(); |
|||
ctx.arc(randomNum(0, width), randomNum(0, height), 1, 0, 2 * Math.PI); |
|||
ctx.closePath(); |
|||
ctx.fillStyle = randomColor(150, 200); |
|||
ctx.fill(); |
|||
} |
|||
return imgCode; |
|||
} |
@ -0,0 +1,48 @@ |
|||
<script lang="ts"> |
|||
export default { |
|||
name: "ReImageVerify" |
|||
}; |
|||
</script> |
|||
|
|||
<script setup lang="ts"> |
|||
import { watch } from "vue"; |
|||
import { useImageVerify } from "./hooks"; |
|||
|
|||
interface Props { |
|||
code?: string; |
|||
} |
|||
|
|||
interface Emits { |
|||
(e: "update:code", code: string): void; |
|||
} |
|||
|
|||
const props = withDefaults(defineProps<Props>(), { |
|||
code: "" |
|||
}); |
|||
|
|||
const emit = defineEmits<Emits>(); |
|||
|
|||
const { domRef, imgCode, setImgCode, getImgCode } = useImageVerify(); |
|||
|
|||
watch( |
|||
() => props.code, |
|||
newValue => { |
|||
setImgCode(newValue); |
|||
} |
|||
); |
|||
watch(imgCode, newValue => { |
|||
emit("update:code", newValue); |
|||
}); |
|||
|
|||
defineExpose({ getImgCode }); |
|||
</script> |
|||
|
|||
<template> |
|||
<canvas |
|||
ref="domRef" |
|||
width="120" |
|||
height="40" |
|||
class="cursor-pointer" |
|||
@click="getImgCode" |
|||
/> |
|||
</template> |
@ -0,0 +1,10 @@ |
|||
import { App } from "vue"; |
|||
import reQrcode from "./src/index"; |
|||
|
|||
export const ReQrcode = Object.assign(reQrcode, { |
|||
install(app: App) { |
|||
app.component(reQrcode.name, reQrcode); |
|||
} |
|||
}); |
|||
|
|||
export default ReQrcode; |
@ -0,0 +1,8 @@ |
|||
.qrcode { |
|||
&--disabled { |
|||
background: rgba(255, 255, 255, 0.95); |
|||
& > div { |
|||
transform: translate(-50%, -50%); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,262 @@ |
|||
import { |
|||
ref, |
|||
unref, |
|||
watch, |
|||
nextTick, |
|||
computed, |
|||
PropType, |
|||
defineComponent |
|||
} from "vue"; |
|||
import "./index.scss"; |
|||
import { isString } from "/@/utils/is"; |
|||
import { cloneDeep } from "lodash-unified"; |
|||
import { propTypes } from "/@/utils/propTypes"; |
|||
import { IconifyIconOffline } from "../../ReIcon"; |
|||
import QRCode, { QRCodeRenderersOptions } from "qrcode"; |
|||
|
|||
interface QrcodeLogo { |
|||
src?: string; |
|||
logoSize?: number; |
|||
bgColor?: string; |
|||
borderSize?: number; |
|||
crossOrigin?: string; |
|||
borderRadius?: number; |
|||
logoRadius?: number; |
|||
} |
|||
|
|||
const props = { |
|||
// img 或者 canvas,img不支持logo嵌套
|
|||
tag: propTypes.string |
|||
.validate((v: string) => ["canvas", "img"].includes(v)) |
|||
.def("canvas"), |
|||
// 二维码内容
|
|||
text: { |
|||
type: [String, Array] as PropType<string | Recordable[]>, |
|||
default: null |
|||
}, |
|||
// qrcode.js配置项
|
|||
options: { |
|||
type: Object as PropType<QRCodeRenderersOptions>, |
|||
default: (): QRCodeRenderersOptions => ({}) |
|||
}, |
|||
// 宽度
|
|||
width: propTypes.number.def(200), |
|||
// logo
|
|||
logo: { |
|||
type: [String, Object] as PropType<Partial<QrcodeLogo> | string>, |
|||
default: (): QrcodeLogo | string => "" |
|||
}, |
|||
// 是否过期
|
|||
disabled: propTypes.bool.def(false), |
|||
// 过期提示内容
|
|||
disabledText: propTypes.string.def("") |
|||
}; |
|||
|
|||
export default defineComponent({ |
|||
name: "ReQrcode", |
|||
props, |
|||
emits: ["done", "click", "disabled-click"], |
|||
setup(props, { emit }) { |
|||
const { toCanvas, toDataURL } = QRCode; |
|||
const loading = ref(true); |
|||
const wrapRef = ref<Nullable<HTMLCanvasElement | HTMLImageElement>>(null); |
|||
const renderText = computed(() => String(props.text)); |
|||
const wrapStyle = computed(() => { |
|||
return { |
|||
width: props.width + "px", |
|||
height: props.width + "px" |
|||
}; |
|||
}); |
|||
const initQrcode = async () => { |
|||
await nextTick(); |
|||
const options = cloneDeep(props.options || {}); |
|||
if (props.tag === "canvas") { |
|||
// 容错率,默认对内容少的二维码采用高容错率,内容多的二维码采用低容错率
|
|||
options.errorCorrectionLevel = |
|||
options.errorCorrectionLevel || |
|||
getErrorCorrectionLevel(unref(renderText)); |
|||
const _width: number = await getOriginWidth(unref(renderText), options); |
|||
options.scale = |
|||
props.width === 0 ? undefined : (props.width / _width) * 4; |
|||
const canvasRef: HTMLCanvasElement = await toCanvas( |
|||
unref(wrapRef) as HTMLCanvasElement, |
|||
unref(renderText), |
|||
options |
|||
); |
|||
if (props.logo) { |
|||
const url = await createLogoCode(canvasRef); |
|||
emit("done", url); |
|||
loading.value = false; |
|||
} else { |
|||
emit("done", canvasRef.toDataURL()); |
|||
loading.value = false; |
|||
} |
|||
} else { |
|||
const url = await toDataURL(renderText.value, { |
|||
errorCorrectionLevel: "H", |
|||
width: props.width, |
|||
...options |
|||
}); |
|||
(unref(wrapRef) as HTMLImageElement).src = url; |
|||
emit("done", url); |
|||
loading.value = false; |
|||
} |
|||
}; |
|||
watch( |
|||
() => renderText.value, |
|||
val => { |
|||
if (!val) return; |
|||
initQrcode(); |
|||
}, |
|||
{ |
|||
deep: true, |
|||
immediate: true |
|||
} |
|||
); |
|||
const createLogoCode = (canvasRef: HTMLCanvasElement) => { |
|||
const canvasWidth = canvasRef.width; |
|||
const logoOptions: QrcodeLogo = Object.assign( |
|||
{ |
|||
logoSize: 0.15, |
|||
bgColor: "#ffffff", |
|||
borderSize: 0.05, |
|||
crossOrigin: "anonymous", |
|||
borderRadius: 8, |
|||
logoRadius: 0 |
|||
}, |
|||
isString(props.logo) ? {} : props.logo |
|||
); |
|||
const { |
|||
logoSize = 0.15, |
|||
bgColor = "#ffffff", |
|||
borderSize = 0.05, |
|||
crossOrigin = "anonymous", |
|||
borderRadius = 8, |
|||
logoRadius = 0 |
|||
} = logoOptions; |
|||
const logoSrc = isString(props.logo) ? props.logo : props.logo.src; |
|||
const logoWidth = canvasWidth * logoSize; |
|||
const logoXY = (canvasWidth * (1 - logoSize)) / 2; |
|||
const logoBgWidth = canvasWidth * (logoSize + borderSize); |
|||
const logoBgXY = (canvasWidth * (1 - logoSize - borderSize)) / 2; |
|||
const ctx = canvasRef.getContext("2d"); |
|||
if (!ctx) return; |
|||
// logo 底色
|
|||
canvasRoundRect(ctx)( |
|||
logoBgXY, |
|||
logoBgXY, |
|||
logoBgWidth, |
|||
logoBgWidth, |
|||
borderRadius |
|||
); |
|||
ctx.fillStyle = bgColor; |
|||
ctx.fill(); |
|||
// logo
|
|||
const image = new Image(); |
|||
if (crossOrigin || logoRadius) { |
|||
image.setAttribute("crossOrigin", crossOrigin); |
|||
} |
|||
(image as any).src = logoSrc; |
|||
// 使用image绘制可以避免某些跨域情况
|
|||
const drawLogoWithImage = (image: HTMLImageElement) => { |
|||
ctx.drawImage(image, logoXY, logoXY, logoWidth, logoWidth); |
|||
}; |
|||
// 使用canvas绘制以获得更多的功能
|
|||
const drawLogoWithCanvas = (image: HTMLImageElement) => { |
|||
const canvasImage = document.createElement("canvas"); |
|||
canvasImage.width = logoXY + logoWidth; |
|||
canvasImage.height = logoXY + logoWidth; |
|||
const imageCanvas = canvasImage.getContext("2d"); |
|||
if (!imageCanvas || !ctx) return; |
|||
imageCanvas.drawImage(image, logoXY, logoXY, logoWidth, logoWidth); |
|||
canvasRoundRect(ctx)(logoXY, logoXY, logoWidth, logoWidth, logoRadius); |
|||
if (!ctx) return; |
|||
const fillStyle = ctx.createPattern(canvasImage, "no-repeat"); |
|||
if (fillStyle) { |
|||
ctx.fillStyle = fillStyle; |
|||
ctx.fill(); |
|||
} |
|||
}; |
|||
// 将 logo绘制到 canvas上
|
|||
return new Promise((resolve: any) => { |
|||
image.onload = () => { |
|||
logoRadius ? drawLogoWithCanvas(image) : drawLogoWithImage(image); |
|||
resolve(canvasRef.toDataURL()); |
|||
}; |
|||
}); |
|||
}; |
|||
// 得到原QrCode的大小,以便缩放得到正确的QrCode大小
|
|||
const getOriginWidth = async ( |
|||
content: string, |
|||
options: QRCodeRenderersOptions |
|||
) => { |
|||
const _canvas = document.createElement("canvas"); |
|||
await toCanvas(_canvas, content, options); |
|||
return _canvas.width; |
|||
}; |
|||
// 对于内容少的QrCode,增大容错率
|
|||
const getErrorCorrectionLevel = (content: string) => { |
|||
if (content.length > 36) { |
|||
return "M"; |
|||
} else if (content.length > 16) { |
|||
return "Q"; |
|||
} else { |
|||
return "H"; |
|||
} |
|||
}; |
|||
// 用于绘制圆角
|
|||
const canvasRoundRect = (ctx: CanvasRenderingContext2D) => { |
|||
return (x: number, y: number, w: number, h: number, r: number) => { |
|||
const minSize = Math.min(w, h); |
|||
if (r > minSize / 2) { |
|||
r = minSize / 2; |
|||
} |
|||
ctx.beginPath(); |
|||
ctx.moveTo(x + r, y); |
|||
ctx.arcTo(x + w, y, x + w, y + h, r); |
|||
ctx.arcTo(x + w, y + h, x, y + h, r); |
|||
ctx.arcTo(x, y + h, x, y, r); |
|||
ctx.arcTo(x, y, x + w, y, r); |
|||
ctx.closePath(); |
|||
return ctx; |
|||
}; |
|||
}; |
|||
const clickCode = () => { |
|||
emit("click"); |
|||
}; |
|||
const disabledClick = () => { |
|||
emit("disabled-click"); |
|||
}; |
|||
return () => ( |
|||
<> |
|||
<div |
|||
v-loading={unref(loading)} |
|||
class="qrcode relative inline-block" |
|||
style={unref(wrapStyle)} |
|||
> |
|||
{props.tag === "canvas" ? ( |
|||
<canvas ref={wrapRef} onClick={clickCode}></canvas> |
|||
) : ( |
|||
<img ref={wrapRef} onClick={clickCode}></img> |
|||
)} |
|||
{props.disabled && ( |
|||
<div |
|||
class="qrcode--disabled absolute top-0 left-0 flex w-full h-full items-center justify-center" |
|||
onClick={disabledClick} |
|||
> |
|||
<div class="absolute top-[50%] left-[50%] font-bold"> |
|||
<IconifyIconOffline |
|||
class="cursor-pointer outline-none" |
|||
icon="refresh-right" |
|||
width="30" |
|||
color="var(--el-color-primary)" |
|||
/> |
|||
<div>{props.disabledText}</div> |
|||
</div> |
|||
</div> |
|||
)} |
|||
</div> |
|||
</> |
|||
); |
|||
} |
|||
}); |
@ -0,0 +1,4 @@ |
|||
import { h, resolveComponent } from "vue"; |
|||
|
|||
export const dynamicComponent = (component: string) => |
|||
h(resolveComponent(component)); |
@ -1,152 +0,0 @@ |
|||
<script setup lang="ts"> |
|||
import { ref } from "vue"; |
|||
import { useRouter } from "vue-router"; |
|||
import { initRouter } from "/@/router/utils"; |
|||
import { storageSession } from "/@/utils/storage"; |
|||
import { addClass, removeClass } from "/@/utils/operate"; |
|||
import bg from "/@/assets/login/bg.png"; |
|||
import avatar from "/@/assets/login/avatar.svg?component"; |
|||
import illustration from "/@/assets/login/illustration.svg?component"; |
|||
|
|||
const router = useRouter(); |
|||
|
|||
let user = ref("admin"); |
|||
let pwd = ref("123456"); |
|||
|
|||
const onLogin = (): void => { |
|||
storageSession.setItem("info", { |
|||
username: "admin", |
|||
accessToken: "eyJhbGciOiJIUzUxMiJ9.test" |
|||
}); |
|||
initRouter("admin").then(() => {}); |
|||
router.push("/"); |
|||
}; |
|||
|
|||
function onUserFocus() { |
|||
addClass(document.querySelector(".user"), "focus"); |
|||
} |
|||
|
|||
function onUserBlur() { |
|||
if (user.value.length === 0) |
|||
removeClass(document.querySelector(".user"), "focus"); |
|||
} |
|||
|
|||
function onPwdFocus() { |
|||
addClass(document.querySelector(".pwd"), "focus"); |
|||
} |
|||
|
|||
function onPwdBlur() { |
|||
if (pwd.value.length === 0) |
|||
removeClass(document.querySelector(".pwd"), "focus"); |
|||
} |
|||
</script> |
|||
|
|||
<template> |
|||
<img :src="bg" class="wave" /> |
|||
<div class="login-container"> |
|||
<div class="img"> |
|||
<illustration /> |
|||
</div> |
|||
<div class="login-box"> |
|||
<div class="login-form"> |
|||
<avatar class="avatar" /> |
|||
<h2 |
|||
v-motion |
|||
:initial="{ |
|||
opacity: 0, |
|||
y: 100 |
|||
}" |
|||
:enter="{ |
|||
opacity: 1, |
|||
y: 0, |
|||
transition: { |
|||
delay: 100 |
|||
} |
|||
}" |
|||
> |
|||
Pure Admin |
|||
</h2> |
|||
<div |
|||
class="input-group user focus" |
|||
v-motion |
|||
:initial="{ |
|||
opacity: 0, |
|||
y: 100 |
|||
}" |
|||
:enter="{ |
|||
opacity: 1, |
|||
y: 0, |
|||
transition: { |
|||
delay: 200 |
|||
} |
|||
}" |
|||
> |
|||
<div class="icon"> |
|||
<IconifyIconOffline icon="user" width="14" height="14" /> |
|||
</div> |
|||
<div> |
|||
<h5>用户名</h5> |
|||
<input |
|||
type="text" |
|||
class="input" |
|||
v-model="user" |
|||
@focus="onUserFocus" |
|||
@blur="onUserBlur" |
|||
/> |
|||
</div> |
|||
</div> |
|||
<div |
|||
class="input-group pwd focus" |
|||
v-motion |
|||
:initial="{ |
|||
opacity: 0, |
|||
y: 100 |
|||
}" |
|||
:enter="{ |
|||
opacity: 1, |
|||
y: 0, |
|||
transition: { |
|||
delay: 300 |
|||
} |
|||
}" |
|||
> |
|||
<div class="icon"> |
|||
<IconifyIconOffline icon="lock" width="14" height="14" /> |
|||
</div> |
|||
<div> |
|||
<h5>密码</h5> |
|||
<input |
|||
type="password" |
|||
class="input" |
|||
v-model="pwd" |
|||
@focus="onPwdFocus" |
|||
@blur="onPwdBlur" |
|||
/> |
|||
</div> |
|||
</div> |
|||
<button |
|||
class="btn" |
|||
v-motion |
|||
:initial="{ |
|||
opacity: 0, |
|||
y: 10 |
|||
}" |
|||
:enter="{ |
|||
opacity: 1, |
|||
y: 0, |
|||
transition: { |
|||
delay: 400 |
|||
} |
|||
}" |
|||
@click="onLogin" |
|||
> |
|||
登录 |
|||
</button> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
</template> |
|||
|
|||
<style scoped> |
|||
@import url("/@/style/login.css"); |
|||
</style> |
@ -0,0 +1,96 @@ |
|||
<script setup lang="ts"> |
|||
import { ref, reactive } from "vue"; |
|||
import Motion from "../utils/motion"; |
|||
import { phoneRules } from "../utils/rule"; |
|||
import { message } from "@pureadmin/components"; |
|||
import type { FormInstance } from "element-plus"; |
|||
import { useVerifyCode } from "../utils/verifyCode"; |
|||
import { useUserStoreHook } from "/@/store/modules/user"; |
|||
import { useRenderIcon } from "/@/components/ReIcon/src/hooks"; |
|||
|
|||
const loading = ref(false); |
|||
const ruleForm = reactive({ |
|||
phone: "", |
|||
verifyCode: "" |
|||
}); |
|||
const ruleFormRef = ref<FormInstance>(); |
|||
const { isDisabled, text } = useVerifyCode(); |
|||
|
|||
const onLogin = async (formEl: FormInstance | undefined) => { |
|||
loading.value = true; |
|||
if (!formEl) return; |
|||
await formEl.validate((valid, fields) => { |
|||
if (valid) { |
|||
// 模拟登陆请求,需根据实际开发进行修改 |
|||
setTimeout(() => { |
|||
message.success("登陆成功"); |
|||
loading.value = false; |
|||
}, 2000); |
|||
} else { |
|||
loading.value = false; |
|||
return fields; |
|||
} |
|||
}); |
|||
}; |
|||
|
|||
function onBack() { |
|||
useVerifyCode().end(); |
|||
useUserStoreHook().SET_CURRENTPAGE(0); |
|||
} |
|||
</script> |
|||
|
|||
<template> |
|||
<el-form ref="ruleFormRef" :model="ruleForm" :rules="phoneRules" size="large"> |
|||
<Motion> |
|||
<el-form-item prop="phone"> |
|||
<el-input |
|||
clearable |
|||
v-model="ruleForm.phone" |
|||
placeholder="手机号码" |
|||
:prefix-icon="useRenderIcon('iphone')" |
|||
/> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="100"> |
|||
<el-form-item prop="verifyCode"> |
|||
<div class="w-full flex justify-between"> |
|||
<el-input |
|||
clearable |
|||
v-model="ruleForm.verifyCode" |
|||
placeholder="短信验证码" |
|||
/> |
|||
<el-button |
|||
:disabled="isDisabled" |
|||
class="ml-2" |
|||
@click="useVerifyCode().start(ruleFormRef, 'phone')" |
|||
> |
|||
{{ text }} |
|||
</el-button> |
|||
</div> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="150"> |
|||
<el-form-item> |
|||
<el-button |
|||
class="w-full" |
|||
size="default" |
|||
type="primary" |
|||
:loading="loading" |
|||
@click="onLogin(ruleFormRef)" |
|||
> |
|||
登陆 |
|||
</el-button> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="200"> |
|||
<el-form-item> |
|||
<el-button class="w-full" size="default" @click="onBack"> |
|||
返回 |
|||
</el-button> |
|||
</el-form-item> |
|||
</Motion> |
|||
</el-form> |
|||
</template> |
@ -0,0 +1,22 @@ |
|||
<script setup lang="ts"> |
|||
import Motion from "../utils/motion"; |
|||
import ReQrcode from "/@/components/ReQrcode"; |
|||
import { useUserStoreHook } from "/@/store/modules/user"; |
|||
</script> |
|||
|
|||
<template> |
|||
<Motion class="-mt-2 -mb-2"> <ReQrcode text="模拟测试" /> </Motion> |
|||
<Motion :delay="100"> |
|||
<el-divider> |
|||
<p class="text-gray-500 text-xs">扫码后点击"确认",即可完成登录</p> |
|||
</el-divider> |
|||
</Motion> |
|||
<Motion :delay="150"> |
|||
<el-button |
|||
class="w-full mt-4" |
|||
@click="useUserStoreHook().SET_CURRENTPAGE(0)" |
|||
> |
|||
返回 |
|||
</el-button> |
|||
</Motion> |
|||
</template> |
@ -0,0 +1,169 @@ |
|||
<script setup lang="ts"> |
|||
import { ref, reactive } from "vue"; |
|||
import Motion from "../utils/motion"; |
|||
import { updateRules } from "../utils/rule"; |
|||
import { message } from "@pureadmin/components"; |
|||
import type { FormInstance } from "element-plus"; |
|||
import { useVerifyCode } from "../utils/verifyCode"; |
|||
import { useUserStoreHook } from "/@/store/modules/user"; |
|||
import { useRenderIcon } from "/@/components/ReIcon/src/hooks"; |
|||
|
|||
const checked = ref(false); |
|||
const loading = ref(false); |
|||
const ruleForm = reactive({ |
|||
username: "", |
|||
phone: "", |
|||
verifyCode: "", |
|||
password: "", |
|||
repeatPassword: "" |
|||
}); |
|||
const ruleFormRef = ref<FormInstance>(); |
|||
const { isDisabled, text } = useVerifyCode(); |
|||
const repeatPasswordRule = [ |
|||
{ |
|||
validator: (rule, value, callback) => { |
|||
if (value === "") { |
|||
callback(new Error("请输入确认密码")); |
|||
} else if (ruleForm.password !== value) { |
|||
callback(new Error("两次密码不一致!")); |
|||
} else { |
|||
callback(); |
|||
} |
|||
}, |
|||
trigger: "blur" |
|||
} |
|||
]; |
|||
|
|||
const onUpdate = async (formEl: FormInstance | undefined) => { |
|||
loading.value = true; |
|||
if (!formEl) return; |
|||
await formEl.validate((valid, fields) => { |
|||
if (valid) { |
|||
if (checked.value) { |
|||
// 模拟请求,需根据实际开发进行修改 |
|||
setTimeout(() => { |
|||
message.success("注册成功"); |
|||
loading.value = false; |
|||
}, 2000); |
|||
} else { |
|||
loading.value = false; |
|||
message.warning("请勾选隐私政策"); |
|||
} |
|||
} else { |
|||
loading.value = false; |
|||
return fields; |
|||
} |
|||
}); |
|||
}; |
|||
|
|||
function onBack() { |
|||
useVerifyCode().end(); |
|||
useUserStoreHook().SET_CURRENTPAGE(0); |
|||
} |
|||
</script> |
|||
|
|||
<template> |
|||
<el-form |
|||
ref="ruleFormRef" |
|||
:model="ruleForm" |
|||
:rules="updateRules" |
|||
size="large" |
|||
> |
|||
<Motion> |
|||
<el-form-item |
|||
:rules="[{ required: true, message: '请输入账号', trigger: 'blur' }]" |
|||
prop="username" |
|||
> |
|||
<el-input |
|||
clearable |
|||
v-model="ruleForm.username" |
|||
placeholder="账号" |
|||
:prefix-icon="useRenderIcon('user')" |
|||
/> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="100"> |
|||
<el-form-item prop="phone"> |
|||
<el-input |
|||
clearable |
|||
v-model="ruleForm.phone" |
|||
placeholder="手机号码" |
|||
:prefix-icon="useRenderIcon('iphone')" |
|||
/> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="150"> |
|||
<el-form-item prop="verifyCode"> |
|||
<div class="w-full flex justify-between"> |
|||
<el-input |
|||
clearable |
|||
v-model="ruleForm.verifyCode" |
|||
placeholder="短信验证码" |
|||
/> |
|||
<el-button |
|||
:disabled="isDisabled" |
|||
class="ml-2" |
|||
@click="useVerifyCode().start(ruleFormRef, 'phone')" |
|||
> |
|||
{{ text }} |
|||
</el-button> |
|||
</div> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="200"> |
|||
<el-form-item prop="password"> |
|||
<el-input |
|||
clearable |
|||
show-password |
|||
v-model="ruleForm.password" |
|||
placeholder="密码" |
|||
:prefix-icon="useRenderIcon('lock')" |
|||
/> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="250"> |
|||
<el-form-item :rules="repeatPasswordRule" prop="repeatPassword"> |
|||
<el-input |
|||
clearable |
|||
show-password |
|||
v-model="ruleForm.repeatPassword" |
|||
placeholder="确认密码" |
|||
:prefix-icon="useRenderIcon('lock')" |
|||
/> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="300"> |
|||
<el-form-item> |
|||
<el-checkbox v-model="checked"> 我已仔细阅读并接受 </el-checkbox> |
|||
<el-button type="text"> 《隐私政策》 </el-button> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="350"> |
|||
<el-form-item> |
|||
<el-button |
|||
class="w-full" |
|||
size="default" |
|||
type="primary" |
|||
:loading="loading" |
|||
@click="onUpdate(ruleFormRef)" |
|||
> |
|||
确定 |
|||
</el-button> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="400"> |
|||
<el-form-item> |
|||
<el-button class="w-full" size="default" @click="onBack"> |
|||
返回 |
|||
</el-button> |
|||
</el-form-item> |
|||
</Motion> |
|||
</el-form> |
|||
</template> |
@ -0,0 +1,141 @@ |
|||
<script setup lang="ts"> |
|||
import { ref, reactive } from "vue"; |
|||
import Motion from "../utils/motion"; |
|||
import { updateRules } from "../utils/rule"; |
|||
import { message } from "@pureadmin/components"; |
|||
import type { FormInstance } from "element-plus"; |
|||
import { useVerifyCode } from "../utils/verifyCode"; |
|||
import { useUserStoreHook } from "/@/store/modules/user"; |
|||
import { useRenderIcon } from "/@/components/ReIcon/src/hooks"; |
|||
|
|||
const loading = ref(false); |
|||
const ruleForm = reactive({ |
|||
phone: "", |
|||
verifyCode: "", |
|||
password: "", |
|||
repeatPassword: "" |
|||
}); |
|||
const ruleFormRef = ref<FormInstance>(); |
|||
const { isDisabled, text } = useVerifyCode(); |
|||
const repeatPasswordRule = [ |
|||
{ |
|||
validator: (rule, value, callback) => { |
|||
if (value === "") { |
|||
callback(new Error("请输入确认密码")); |
|||
} else if (ruleForm.password !== value) { |
|||
callback(new Error("两次密码不一致!")); |
|||
} else { |
|||
callback(); |
|||
} |
|||
}, |
|||
trigger: "blur" |
|||
} |
|||
]; |
|||
|
|||
const onUpdate = async (formEl: FormInstance | undefined) => { |
|||
loading.value = true; |
|||
if (!formEl) return; |
|||
await formEl.validate((valid, fields) => { |
|||
if (valid) { |
|||
// 模拟请求,需根据实际开发进行修改 |
|||
setTimeout(() => { |
|||
message.success("修改密码成功"); |
|||
loading.value = false; |
|||
}, 2000); |
|||
} else { |
|||
loading.value = false; |
|||
return fields; |
|||
} |
|||
}); |
|||
}; |
|||
|
|||
function onBack() { |
|||
useVerifyCode().end(); |
|||
useUserStoreHook().SET_CURRENTPAGE(0); |
|||
} |
|||
</script> |
|||
|
|||
<template> |
|||
<el-form |
|||
ref="ruleFormRef" |
|||
:model="ruleForm" |
|||
:rules="updateRules" |
|||
size="large" |
|||
> |
|||
<Motion> |
|||
<el-form-item prop="phone"> |
|||
<el-input |
|||
clearable |
|||
v-model="ruleForm.phone" |
|||
placeholder="手机号码" |
|||
:prefix-icon="useRenderIcon('iphone')" |
|||
/> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="100"> |
|||
<el-form-item prop="verifyCode"> |
|||
<div class="w-full flex justify-between"> |
|||
<el-input |
|||
clearable |
|||
v-model="ruleForm.verifyCode" |
|||
placeholder="短信验证码" |
|||
/> |
|||
<el-button |
|||
:disabled="isDisabled" |
|||
class="ml-2" |
|||
@click="useVerifyCode().start(ruleFormRef, 'phone')" |
|||
> |
|||
{{ text }} |
|||
</el-button> |
|||
</div> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="150"> |
|||
<el-form-item prop="password"> |
|||
<el-input |
|||
clearable |
|||
show-password |
|||
v-model="ruleForm.password" |
|||
placeholder="密码" |
|||
:prefix-icon="useRenderIcon('lock')" |
|||
/> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="200"> |
|||
<el-form-item :rules="repeatPasswordRule" prop="repeatPassword"> |
|||
<el-input |
|||
clearable |
|||
show-password |
|||
v-model="ruleForm.repeatPassword" |
|||
placeholder="确认密码" |
|||
:prefix-icon="useRenderIcon('lock')" |
|||
/> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="250"> |
|||
<el-form-item> |
|||
<el-button |
|||
class="w-full" |
|||
size="default" |
|||
type="primary" |
|||
:loading="loading" |
|||
@click="onUpdate(ruleFormRef)" |
|||
> |
|||
确定 |
|||
</el-button> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="300"> |
|||
<el-form-item> |
|||
<el-button class="w-full" size="default" @click="onBack"> |
|||
返回 |
|||
</el-button> |
|||
</el-form-item> |
|||
</Motion> |
|||
</el-form> |
|||
</template> |
@ -0,0 +1,208 @@ |
|||
<script setup lang="ts"> |
|||
import Motion from "./utils/motion"; |
|||
import { useRouter } from "vue-router"; |
|||
import { loginRules } from "./utils/rule"; |
|||
import phone from "./components/phone.vue"; |
|||
import qrCode from "./components/qrCode.vue"; |
|||
import regist from "./components/regist.vue"; |
|||
import update from "./components/update.vue"; |
|||
import { initRouter } from "/@/router/utils"; |
|||
import { message } from "@pureadmin/components"; |
|||
import type { FormInstance } from "element-plus"; |
|||
import { storageSession } from "/@/utils/storage"; |
|||
import { ref, reactive, watch, computed } from "vue"; |
|||
import { operates, thirdParty } from "./utils/enums"; |
|||
import { useUserStoreHook } from "/@/store/modules/user"; |
|||
import { bg, avatar, currentWeek } from "./utils/static"; |
|||
import { ReImageVerify } from "/@/components/ReImageVerify"; |
|||
import { useRenderIcon } from "/@/components/ReIcon/src/hooks"; |
|||
|
|||
const imgCode = ref(""); |
|||
const router = useRouter(); |
|||
const loading = ref(false); |
|||
const checked = ref(false); |
|||
const ruleFormRef = ref<FormInstance>(); |
|||
const currentPage = computed(() => { |
|||
return useUserStoreHook().currentPage; |
|||
}); |
|||
|
|||
const ruleForm = reactive({ |
|||
username: "admin", |
|||
password: "admin123", |
|||
verifyCode: "" |
|||
}); |
|||
|
|||
const onLogin = async (formEl: FormInstance | undefined) => { |
|||
loading.value = true; |
|||
if (!formEl) return; |
|||
await formEl.validate((valid, fields) => { |
|||
if (valid) { |
|||
// 模拟请求,需根据实际开发进行修改 |
|||
setTimeout(() => { |
|||
loading.value = false; |
|||
storageSession.setItem("info", { |
|||
username: "admin", |
|||
accessToken: "eyJhbGciOiJIUzUxMiJ9.test" |
|||
}); |
|||
initRouter("admin").then(() => {}); |
|||
message.success("登陆成功"); |
|||
router.push("/"); |
|||
}, 2000); |
|||
} else { |
|||
loading.value = false; |
|||
return fields; |
|||
} |
|||
}); |
|||
}; |
|||
|
|||
function onHandle(value) { |
|||
useUserStoreHook().SET_CURRENTPAGE(value); |
|||
} |
|||
|
|||
watch(imgCode, value => { |
|||
useUserStoreHook().SET_VERIFYCODE(value); |
|||
}); |
|||
</script> |
|||
|
|||
<template> |
|||
<img :src="bg" class="wave" /> |
|||
<div class="login-container"> |
|||
<div class="img"> |
|||
<component :is="currentWeek" /> |
|||
</div> |
|||
<div class="login-box"> |
|||
<div class="login-form"> |
|||
<avatar class="avatar" /> |
|||
<Motion> |
|||
<h2>Pure Admin</h2> |
|||
</Motion> |
|||
|
|||
<el-form |
|||
v-if="currentPage === 0" |
|||
ref="ruleFormRef" |
|||
:model="ruleForm" |
|||
:rules="loginRules" |
|||
size="large" |
|||
> |
|||
<Motion :delay="100"> |
|||
<el-form-item prop="username"> |
|||
<el-input |
|||
clearable |
|||
:input-style="{ 'user-select': 'none' }" |
|||
v-model="ruleForm.username" |
|||
placeholder="账号" |
|||
:prefix-icon="useRenderIcon('user')" |
|||
/> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="150"> |
|||
<el-form-item prop="password"> |
|||
<el-input |
|||
clearable |
|||
:input-style="{ 'user-select': 'none' }" |
|||
show-password |
|||
v-model="ruleForm.password" |
|||
placeholder="密码" |
|||
:prefix-icon="useRenderIcon('lock')" |
|||
/> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="200"> |
|||
<el-form-item prop="verifyCode"> |
|||
<el-input |
|||
clearable |
|||
:input-style="{ 'user-select': 'none' }" |
|||
v-model="ruleForm.verifyCode" |
|||
placeholder="验证码" |
|||
> |
|||
<template v-slot:append> |
|||
<ReImageVerify v-model:code="imgCode" /> |
|||
</template> |
|||
</el-input> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="250"> |
|||
<el-form-item> |
|||
<div class="w-full h-20px flex justify-between items-center"> |
|||
<el-checkbox v-model="checked">记住密码</el-checkbox> |
|||
<el-button |
|||
type="text" |
|||
@click="useUserStoreHook().SET_CURRENTPAGE(4)" |
|||
> |
|||
忘记密码? |
|||
</el-button> |
|||
</div> |
|||
<el-button |
|||
class="w-full mt-4" |
|||
size="default" |
|||
type="primary" |
|||
:loading="loading" |
|||
@click="onLogin(ruleFormRef)" |
|||
> |
|||
登录 |
|||
</el-button> |
|||
</el-form-item> |
|||
</Motion> |
|||
|
|||
<Motion :delay="300"> |
|||
<el-form-item> |
|||
<div class="w-full h-20px flex justify-between items-center"> |
|||
<el-button |
|||
v-for="(item, index) in operates" |
|||
:key="index" |
|||
class="w-full mt-4" |
|||
size="default" |
|||
@click="onHandle(index + 1)" |
|||
> |
|||
{{ item.title }} |
|||
</el-button> |
|||
</div> |
|||
</el-form-item> |
|||
</Motion> |
|||
</el-form> |
|||
|
|||
<Motion v-if="currentPage === 0" :delay="350"> |
|||
<el-form-item> |
|||
<el-divider> |
|||
<p class="text-gray-500 text-xs">第三方登录</p> |
|||
</el-divider> |
|||
<div class="w-full flex justify-evenly"> |
|||
<span |
|||
v-for="(item, index) in thirdParty" |
|||
:key="index" |
|||
:title="`${item.title}登陆`" |
|||
> |
|||
<IconifyIconOnline |
|||
:icon="`ri:${item.icon}-fill`" |
|||
width="20" |
|||
class="cursor-pointer text-gray-500 hover:text-blue-400" |
|||
/> |
|||
</span> |
|||
</div> |
|||
</el-form-item> |
|||
</Motion> |
|||
<!-- 手机号登陆 --> |
|||
<phone v-if="currentPage === 1" /> |
|||
<!-- 二维码登陆 --> |
|||
<qrCode v-if="currentPage === 2" /> |
|||
<!-- 注册 --> |
|||
<regist v-if="currentPage === 3" /> |
|||
<!-- 忘记密码 --> |
|||
<update v-if="currentPage === 4" /> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
</template> |
|||
|
|||
<style scoped> |
|||
@import url("/@/style/login.css"); |
|||
</style> |
|||
|
|||
<style lang="scss" scoped> |
|||
:deep(.el-input-group__append, .el-input-group__prepend) { |
|||
padding: 0; |
|||
} |
|||
</style> |
@ -0,0 +1,32 @@ |
|||
const operates = [ |
|||
{ |
|||
title: "手机登录" |
|||
}, |
|||
{ |
|||
title: "二维码登录" |
|||
}, |
|||
{ |
|||
title: "注册" |
|||
} |
|||
]; |
|||
|
|||
const thirdParty = [ |
|||
{ |
|||
title: "微信", |
|||
icon: "wechat" |
|||
}, |
|||
{ |
|||
title: "支付宝", |
|||
icon: "alipay" |
|||
}, |
|||
{ |
|||
title: "QQ", |
|||
icon: "qq" |
|||
}, |
|||
{ |
|||
title: "微博", |
|||
icon: "weibo" |
|||
} |
|||
]; |
|||
|
|||
export { operates, thirdParty }; |
@ -0,0 +1,40 @@ |
|||
import { h, defineComponent, withDirectives, resolveDirective } from "vue"; |
|||
|
|||
// 封装@vueuse/motion动画库中的自定义指令v-motion
|
|||
export default defineComponent({ |
|||
name: "Motion", |
|||
props: { |
|||
delay: { |
|||
type: Number, |
|||
default: 50 |
|||
} |
|||
}, |
|||
render() { |
|||
const { delay } = this; |
|||
const motion = resolveDirective("motion"); |
|||
return withDirectives( |
|||
h( |
|||
"div", |
|||
{}, |
|||
{ |
|||
default: () => [this.$slots.default()] |
|||
} |
|||
), |
|||
[ |
|||
[ |
|||
motion, |
|||
{ |
|||
initial: { opacity: 0, y: 100 }, |
|||
enter: { |
|||
opacity: 1, |
|||
y: 0, |
|||
transition: { |
|||
delay |
|||
} |
|||
} |
|||
} |
|||
] |
|||
] |
|||
); |
|||
} |
|||
}); |
@ -0,0 +1,128 @@ |
|||
import { reactive } from "vue"; |
|||
import { isPhone } from "/@/utils/is"; |
|||
import type { FormRules } from "element-plus"; |
|||
import { useUserStoreHook } from "/@/store/modules/user"; |
|||
|
|||
/** 6位数字验证码正则 */ |
|||
export const REGEXP_SIX = /^\d{6}$/; |
|||
|
|||
/** 密码正则(密码格式应为8-18位数字、字母、符号的任意两种组合) */ |
|||
export const REGEXP_PWD = |
|||
/^(?![0-9]+$)(?![a-z]+$)(?![A-Z]+$)(?!([^(0-9a-zA-Z)]|[()])+$)(?!^.*[\u4E00-\u9FA5].*$)([^(0-9a-zA-Z)]|[()]|[a-z]|[A-Z]|[0-9]){8,18}$/; |
|||
|
|||
/** 登陆校验 */ |
|||
const loginRules = reactive(<FormRules>{ |
|||
username: [{ required: true, message: "请输入账号", trigger: "blur" }], |
|||
password: [ |
|||
{ |
|||
validator: (rule, value, callback) => { |
|||
if (value === "") { |
|||
callback(new Error("请输入密码")); |
|||
} else if (!REGEXP_PWD.test(value)) { |
|||
callback( |
|||
new Error("密码格式应为8-18位数字、字母、符号的任意两种组合") |
|||
); |
|||
} else { |
|||
callback(); |
|||
} |
|||
}, |
|||
trigger: "blur" |
|||
} |
|||
], |
|||
verifyCode: [ |
|||
{ |
|||
validator: (rule, value, callback) => { |
|||
if (value === "") { |
|||
callback(new Error("请输入验证码")); |
|||
} else if (useUserStoreHook().verifyCode !== value) { |
|||
callback(new Error("请输入正确的验证码")); |
|||
} else { |
|||
callback(); |
|||
} |
|||
}, |
|||
trigger: "blur" |
|||
} |
|||
] |
|||
}); |
|||
|
|||
/** 手机登陆校验 */ |
|||
const phoneRules = reactive(<FormRules>{ |
|||
phone: [ |
|||
{ |
|||
validator: (rule, value, callback) => { |
|||
if (value === "") { |
|||
callback(new Error("请输入手机号码")); |
|||
} else if (!isPhone(value)) { |
|||
callback(new Error("请输入正确的手机号码格式")); |
|||
} else { |
|||
callback(); |
|||
} |
|||
}, |
|||
trigger: "blur" |
|||
} |
|||
], |
|||
verifyCode: [ |
|||
{ |
|||
validator: (rule, value, callback) => { |
|||
if (value === "") { |
|||
callback(new Error("请输入验证码")); |
|||
} else if (!REGEXP_SIX.test(value)) { |
|||
callback(new Error("请输入6位数字验证码")); |
|||
} else { |
|||
callback(); |
|||
} |
|||
}, |
|||
trigger: "blur" |
|||
} |
|||
] |
|||
}); |
|||
|
|||
/** 忘记密码校验 */ |
|||
const updateRules = reactive(<FormRules>{ |
|||
phone: [ |
|||
{ |
|||
validator: (rule, value, callback) => { |
|||
if (value === "") { |
|||
callback(new Error("请输入手机号码")); |
|||
} else if (!isPhone(value)) { |
|||
callback(new Error("请输入正确的手机号码格式")); |
|||
} else { |
|||
callback(); |
|||
} |
|||
}, |
|||
trigger: "blur" |
|||
} |
|||
], |
|||
verifyCode: [ |
|||
{ |
|||
validator: (rule, value, callback) => { |
|||
if (value === "") { |
|||
callback(new Error("请输入验证码")); |
|||
} else if (!REGEXP_SIX.test(value)) { |
|||
callback(new Error("请输入6位数字验证码")); |
|||
} else { |
|||
callback(); |
|||
} |
|||
}, |
|||
trigger: "blur" |
|||
} |
|||
], |
|||
password: [ |
|||
{ |
|||
validator: (rule, value, callback) => { |
|||
if (value === "") { |
|||
callback(new Error("请输入密码")); |
|||
} else if (!REGEXP_PWD.test(value)) { |
|||
callback( |
|||
new Error("密码格式应为8-18位数字、字母、符号的任意两种组合") |
|||
); |
|||
} else { |
|||
callback(); |
|||
} |
|||
}, |
|||
trigger: "blur" |
|||
} |
|||
] |
|||
}); |
|||
|
|||
export { loginRules, phoneRules, updateRules }; |
@ -0,0 +1,10 @@ |
|||
import { computed } from "vue"; |
|||
import bg from "/@/assets/login/bg.png"; |
|||
import avatar from "/@/assets/login/avatar.svg?component"; |
|||
import illustration from "/@/assets/login/illustration.svg?component"; |
|||
|
|||
const currentWeek = computed(() => { |
|||
return illustration; |
|||
}); |
|||
|
|||
export { bg, avatar, currentWeek }; |
@ -0,0 +1,50 @@ |
|||
import type { FormInstance, FormItemProp } from "element-plus"; |
|||
import { cloneDeep } from "lodash-unified"; |
|||
import { ref } from "vue"; |
|||
|
|||
const isDisabled = ref(false); |
|||
const TEXT = "获取验证码"; |
|||
const timer = ref(null); |
|||
const text = ref(TEXT); |
|||
|
|||
export const useVerifyCode = () => { |
|||
const start = async ( |
|||
formEl: FormInstance | undefined, |
|||
props: FormItemProp, |
|||
time = 60 |
|||
) => { |
|||
if (!formEl) return; |
|||
const initTime = cloneDeep(time); |
|||
await formEl.validateField(props, isValid => { |
|||
if (isValid) { |
|||
clearInterval(timer.value); |
|||
timer.value = setInterval(() => { |
|||
if (time > 0) { |
|||
text.value = `${time}秒后重新获取`; |
|||
isDisabled.value = true; |
|||
time -= 1; |
|||
} else { |
|||
text.value = TEXT; |
|||
isDisabled.value = false; |
|||
clearInterval(timer.value); |
|||
time = initTime; |
|||
} |
|||
}, 1000); |
|||
} |
|||
}); |
|||
}; |
|||
|
|||
const end = () => { |
|||
text.value = TEXT; |
|||
isDisabled.value = false; |
|||
clearInterval(timer.value); |
|||
}; |
|||
|
|||
return { |
|||
isDisabled, |
|||
timer, |
|||
text, |
|||
start, |
|||
end |
|||
}; |
|||
}; |
Write
Preview
Loading…
Cancel
Save
Reference in new issue